Remove or Delete File - Python os.remove() - Examples
Python - Delete File
To remove or delete a file using Python, call the remove()
method of the os
library with the path to the file passed as an argument to the function.
In this tutorial, we will learn how to delete a file and explore different scenarios that may occur during the deletion process, such as handling missing files.
Examples
1. Remove a Given File
This example demonstrates how to delete a file named data.txt
, located in the same directory as the program.
Python Program
import os
os.remove('data.txt')
print('The file is removed.')
Explanation (Step-by-Step):
- Import the
os
module, which provides functions to interact with the file system. - Call
os.remove('data.txt')
to delete the file nameddata.txt
from the current working directory. - If the file exists, it will be removed, and the program will continue to execute the next line.
- Print the message
'The file is removed.'
to indicate successful deletion.
If the file is located in a different directory, specify the absolute path to the file:
Python Program
import os
os.remove('C:\workspace\python\data.txt')
print('The file is removed.')
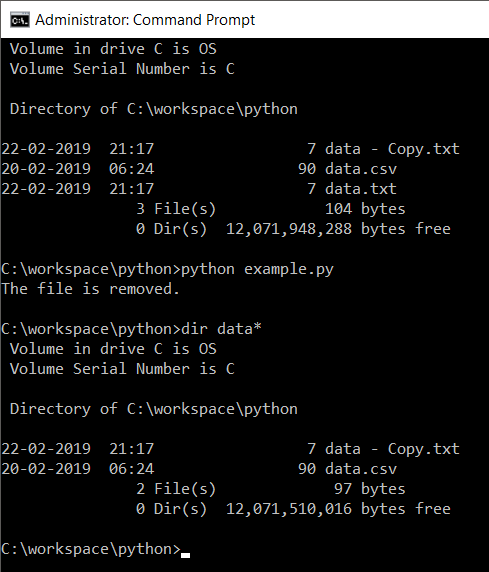
Explanation (Step-by-Step):
- Import the
os
module. - Use
os.remove()
with the absolute file path'C:\workspace\python\data.txt'
to delete the file located in a specific directory. - If the file exists at the specified path, it will be deleted.
- Print the message
'The file is removed.'
to confirm the operation.
2. Try to Remove a File That Does Not Exist
This example shows the error raised when attempting to delete a file that does not exist at the specified path.
Python Program
import os
os.remove('C:\workspace\python\aslkdjfa.txt')
print('The file is removed.')
Output
FileNotFoundError: [WinError 2] The system cannot find the file specified: 'aslkdjfa.txt'
Explanation (Step-by-Step):
- Import the
os
module. - Attempt to delete a file named
'aslkdjfa.txt'
located at the path'C:\workspace\python\'
usingos.remove()
. - Since the file does not exist, Python raises a
FileNotFoundError
. - The error includes details such as the error code
[WinError 2]
and the path of the missing file. - To avoid this error, check if the file exists using
os.path.exists()
before callingos.remove()
.
Summary
In this tutorial, we explored how to delete a file using the os
library's remove()
function in Python, including scenarios where the file exists or does not exist.