Arrays in C++
In this tutorial, we will learn about arrays in C++. We will cover the basics of declaring and using arrays, including accessing and modifying elements.
What is an Array
An array is a collection of elements of the same type stored in contiguous memory locations. It allows you to store multiple items under a single name and access them using an index.
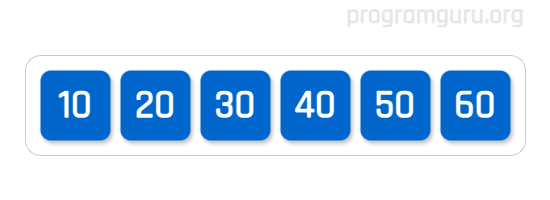
Syntax
The syntax to declare an array in C++ is:
type arrayName[arraySize];
Working with Arrays
We will learn how to declare, initialize, access, and modify arrays in C++.
Example 1: Declaring and Initializing an Array
- Declare an integer array named
arr
with 5 elements. - Initialize the array with values {1, 2, 3, 4, 5}.
- Print all the elements of the array.
C++ Program
#include <iostream>
using namespace std;
int main() {
int arr[5] = {1, 2, 3, 4, 5};
for (int i = 0; i < 5; i++) {
cout << arr[i] << " ";
}
return 0;
}
Output
1 2 3 4 5
Example 2: Accessing Array Elements
- Declare an integer array named
arr
with 5 elements. - Initialize the array with values {10, 20, 30, 40, 50}.
- Access and print the third element of the array.
C++ Program
#include <iostream>
using namespace std;
int main() {
int arr[5] = {10, 20, 30, 40, 50};
cout << "The third element is: " << arr[2] << endl;
return 0;
}
Output
The third element is: 30
Example 3: Modifying Array Elements
- Declare an integer array named
arr
with 5 elements. - Initialize the array with values {5, 10, 15, 20, 25}.
- Modify the second element of the array to 50.
- Print all the elements of the array.
C++ Program
#include <iostream>
using namespace std;
int main() {
int arr[5] = {5, 10, 15, 20, 25};
arr[1] = 50;
for (int i = 0; i < 5; i++) {
cout << arr[i] << " ";
}
return 0;
}
Output
5 50 15 20 25