Arrays in Dart
In this tutorial, we will learn about arrays in Dart. We will cover the basics of declaring and using arrays, including accessing and modifying elements.
What is an Array
An array, known as a List in Dart, is a collection of elements of the same type stored in contiguous memory locations. It allows you to store multiple items under a single name and access them using an index.
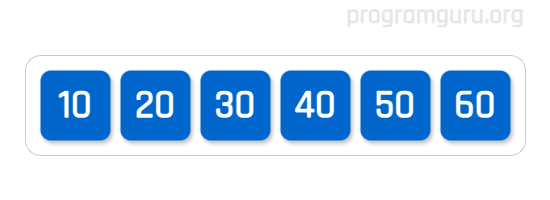
Syntax
The syntax to declare an array in Dart is:
List<type> listName = [elements];
Working with Arrays
We will learn how to declare, initialize, access, and modify arrays in Dart.
Example 1: Declaring and Initializing an Array
- Declare an integer list named
arr
with 5 elements. - Initialize the list with values [1, 2, 3, 4, 5].
- Print all the elements of the list.
Dart Program
void main() {
List<int> arr = [1, 2, 3, 4, 5];
for (int num in arr) {
print(num);
}
}
Output
1 2 3 4 5
Example 2: Accessing Array Elements
- Declare an integer list named
arr
with 5 elements. - Initialize the list with values [10, 20, 30, 40, 50].
- Access and print the third element of the list.
Dart Program
void main() {
List<int> arr = [10, 20, 30, 40, 50];
print('The third element is: ' + arr[2].toString());
}
Output
The third element is: 30
Example 3: Modifying Array Elements
- Declare an integer list named
arr
with 5 elements. - Initialize the list with values [5, 10, 15, 20, 25].
- Modify the second element of the list to 50.
- Print all the elements of the list.
Dart Program
void main() {
List<int> arr = [5, 10, 15, 20, 25];
arr[1] = 50;
for (int num in arr) {
print(num);
}
}
Output
5 50 15 20 25