If Statement in R
In this tutorial, we will learn about if statements in R. We will cover the basics of conditional execution using if statements.
What is an If statement
An if statement is a conditional statement that executes a block of code if a specified condition is true.
Syntax
The syntax for the if statement in R is:
if (condition) {
# Code block to execute if condition is true
}
The if statement evaluates the specified condition. If the condition is true, the code block inside the if statement is executed; otherwise, it is skipped.
The following is the flowchart of how execution flows from start to the end of an if statement.
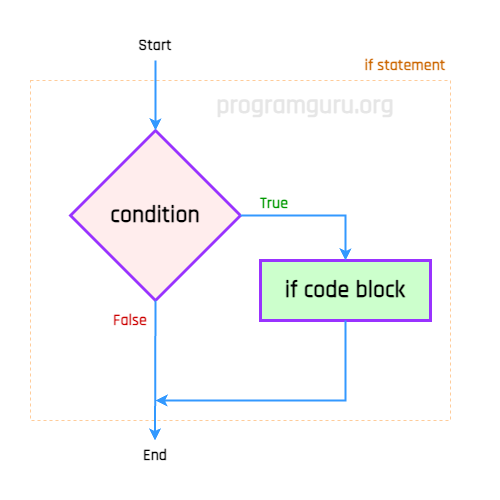
Example 1: Checking if a Number is Even
In R, checking if a number is even can be done using the modulo operator (%), where the remainder when divided by 2 is zero.
For example,
- Declare a variable
num
. - Assign a value to
num
. - Use an if statement to check if
num
is even. - Print a message indicating whether
num
is even or not.
R Program
num <- 10
if (num %% 2 == 0) {
print(paste(num, 'is even.'))
}
Output
[1] "10 is even."
Example 2: Checking if a String Starts with a Specific Value
In R, checking if a string starts with a specific value can be done using the startsWith()
function. The function returns a boolean value, which can be used as a condition in the if statement.
For example,
- Declare a variable
str
. - Assign a value to
str
. - Use an if statement to check if
str
starts with a specific value. - Print a message indicating the result of the check.
R Program
str <- 'Hello, world!'
if (startsWith(str, 'Hello')) {
print('String starts with "Hello".')
}
Output
[1] "String starts with \"Hello\"."
Example 3: Checking if a Number is Positive
In R, checking if a number is positive within an if statement involves evaluating the condition where the number is greater than zero using the greater than operator (>).
For example,
- Declare a variable
num
. - Assign a value to
num
. - Use an if statement to check if
num
is positive. - Print a message indicating whether
num
is positive or not.
R Program
num <- -5
if (num > 0) {
print(paste(num, 'is positive.'))
}