Loops in Swift
In this tutorial, we will learn about different types of loops in Swift. We will cover the basics of `for-in`, `while`, and `repeat-while` loops.
What is a Loop
A loop is a control flow statement that allows code to be executed repeatedly based on a condition.
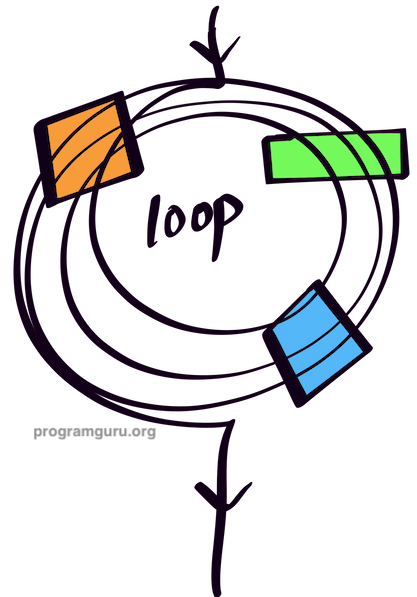
Swift supports three types of loops: `for-in` loops, `while` loops, and `repeat-while` loops.
For-In Loop
A `for-in` loop is used when iterating over a sequence, such as arrays, ranges, or strings. The syntax for the for-in loop is:
for item in sequence {
// Code block to be executed
}
While Loop
A `while` loop is used when the number of iterations is not known beforehand. The syntax for the while loop is:
while condition {
// Code block to be executed
}
Repeat-While Loop
A `repeat-while` loop is similar to a `do-while` loop in other languages, where the code block is executed at least once before checking the condition. The syntax for the repeat-while loop is:
repeat {
// Code block to be executed
} while condition
Example 1: Printing Numbers from 1 to 5 using For-In Loop
- Use a for-in loop to print numbers from 1 to 5.
Swift Program
for i in 1...5 {
print(i, terminator: " ")
}
Output
1 2 3 4 5
Example 2: Printing Numbers from 1 to 3 using While Loop
- Declare an integer variable
i
and initialize it to 1. - Use a while loop to print numbers from 1 to 3.
Swift Program
var i = 1
while i <= 3 {
print(i, terminator: " ")
i += 1
}
Output
1 2 3
Example 3: Printing Numbers from 1 to 3 using Repeat-While Loop
- Declare an integer variable
i
and initialize it to 1. - Use a repeat-while loop to print numbers from 1 to 3.
Swift Program
var i = 1
repeat {
print(i, terminator: " ")
i += 1
} while i <= 3
Output
1 2 3