How to find Average of Two Numbers in C++ - Step by Step Examples
How to find Average of Two Numbers in C++ ?
Answer
To find the average of two numbers in C++, you can add the numbers together and divide by 2.
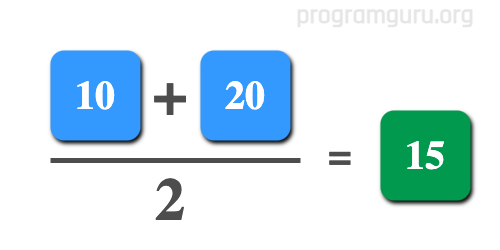
✐ Examples
1 Average of Two Numbers
In this example,
- We declare variables
num1
andnum2
and assign values to them. - We add
num1
andnum2
together and divide by 2 to get the average. - We print the average using
cout
stream.
C++ Program
#include <iostream>
using namespace std;
int main() {
int num1 = 10;
int num2 = 20;
float average = (num1 + num2) / 2.0;
cout << 'Average of ' << num1 << ' and ' << num2 << ' is: ' << average << endl;
return 0;
}
Output
Average of 10 and 20 is: 15
Summary
In this tutorial, we learned How to find Average of Two Numbers in C++ language with well detailed examples.