Loops in Go
In this tutorial, we will learn about different types of loops in Go. We will cover the basics of for loops, which is the only loop type in Go that can be used in various forms to achieve similar functionalities as while and do-while loops in other languages.
What is a Loop
A loop is a control flow statement that allows code to be executed repeatedly based on a condition.
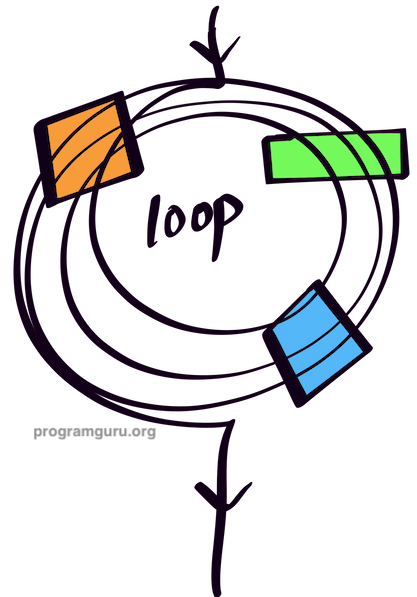
Go supports only the for loop, but it can be used in different forms to create other loop types.
For Loop
A for loop is used when the number of iterations is known before entering the loop. The syntax for the for loop is:
for initialization; condition; post {
// Code block to be executed
}
For Loop as While Loop
A for loop can be used as a while loop by omitting the initialization and post statements. The syntax is:
for condition {
// Code block to be executed
}
For Loop as Do-While Loop
A for loop can be used as a do-while loop by using a for loop with a break statement. The syntax is:
for {
// Code block to be executed
if condition {
break
}
}
Example 1: Printing Numbers from 1 to 10 using For Loop
- Declare an integer variable
i
. - Use a for loop to print numbers from 1 to 10.
Go Program
package main
import "fmt"
func main() {
for i := 1; i <= 10; i++ {
fmt.Print(i, " ")
}
}
Output
1 2 3 4 5 6 7 8 9 10
Example 2: Printing Numbers from 1 to 5 using For Loop as While Loop
- Declare an integer variable
i
and initialize it to 1. - Use a for loop to print numbers from 1 to 5.
Go Program
package main
import "fmt"
func main() {
i := 1
for i <= 5 {
fmt.Print(i, " ")
i++
}
}
Output
1 2 3 4 5
Example 3: Printing Numbers from 1 to 3 using For Loop as Do-While Loop
- Declare an integer variable
i
and initialize it to 1. - Use a for loop with a break statement to print numbers from 1 to 3.
Go Program
package main
import "fmt"
func main() {
i := 1
for {
fmt.Print(i, " ")
i++
if i > 3 {
break
}
}
}
Output
1 2 3