How to find Average of Three Numbers in Java - Step by Step Examples
How to find Average of Three Numbers in Java ?
Answer
To find the average of three numbers in Java, you can add the three numbers together and then divide the sum by 3.
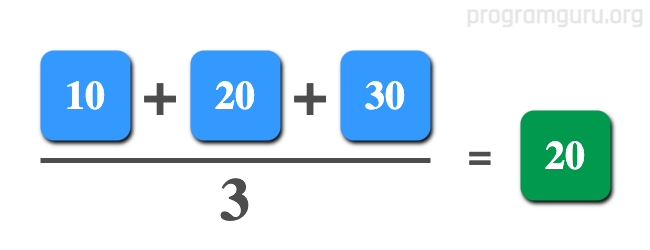
✐ Examples
1 Find Average of Three Integers
In this example,
- We create three integer variables named
num1
,num2
, andnum3
and assign them values. - We calculate the sum of these three integers and store the result in a variable named
sum
. - We find the average by dividing the
sum
by 3.0 (to get a floating-point result) and store it in a variable namedaverage
. - Finally, we print the average to the standard output.
Java Program
public class Main {
public static void main(String[] args) {
int num1 = 5;
int num2 = 10;
int num3 = 15;
int sum = num1 + num2 + num3;
double average = sum / 3.0;
System.out.println("The average is: " + average);
}
}
Output
The average is: 10.0
2 Find Average of Three Floating-Point Numbers
In this example,
- We create three double variables named
num1
,num2
, andnum3
and assign them values. - We calculate the sum of these three doubles and store the result in a variable named
sum
. - We find the average by dividing the
sum
by 3 and store it in a variable namedaverage
. - Finally, we print the average to the standard output.
Java Program
public class Main {
public static void main(String[] args) {
double num1 = 5.5;
double num2 = 10.5;
double num3 = 15.5;
double sum = num1 + num2 + num3;
double average = sum / 3;
System.out.println("The average is: " + average);
}
}
Output
The average is: 10.5
Summary
In this tutorial, we learned How to find Average of Three Numbers in Java language with well detailed examples.