Python BeautifulSoup - Get class of HTML Element
Python BeautifulSoup - Get class of HTML Element
To get the class attribute of a HTML element in Python using BeautifulSoup, you can use Tag.attrs property. The attrs property returns a dictionary with attribute names as keys, and the attribute values as respective values for the keys. You can get the value of class attribute from this dictionary. The value for class attribute is returned as a list of class names.
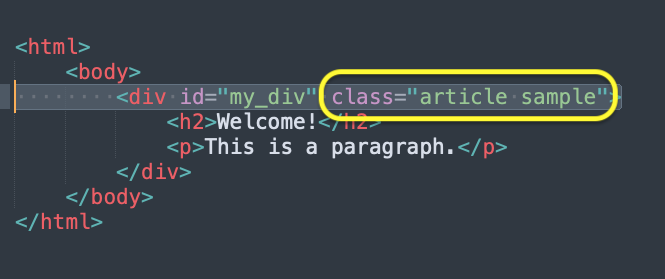
1. Get class attribute of a Div element using attrs property in Python
In the following program, we take a sample HTML content in html_content variable, find the first div element, and then get the class attribute of this div element using attrs property.
Python Program
from bs4 import BeautifulSoup
# Sample HTML content
html_content = """
<html>
<body>
<div id="my_div" class="article sample">
<h2>Welcome!</h2>
<p>This is a paragraph.</p>
</div>
</body>
</html>
"""
# Parse the HTML content
soup = BeautifulSoup(html_content, 'html.parser')
# Consider this as given element
element = soup.find_all("div")[0]
# Get attributes of element
attributes = element.attrs
# Get class from attributes
attr_class = attributes['class']
print(f"class value : {attr_class}")
Output
class value : ['article', 'sample']
The class attribute of the element <div id="my_div" class="article sample">
is a list of 'article'
and 'sample'
values.
Summary
In this Python BeautifulSoup tutorial, given the HTML element, we have seen how to get the class attribute of given HTML element using Tag.attrs property.