Python phonenumbers library
The Python phonenumbers library is used for validating, formatting, and parsing international phone numbers using the Google’s libphonenumber library.
The library supports phone number geocoding, carrier detection, and timezone detection. It is widely used in various application such as e-commerce, customer service, and telecommunication.
In this tutorial, we will learn how to install this phonenumbers library using pip, and different use cases with examples.
Install phonenumbers
To install phonenumbers library via pip, run the following command in terminal or command prompt.
pip install phonenumbers
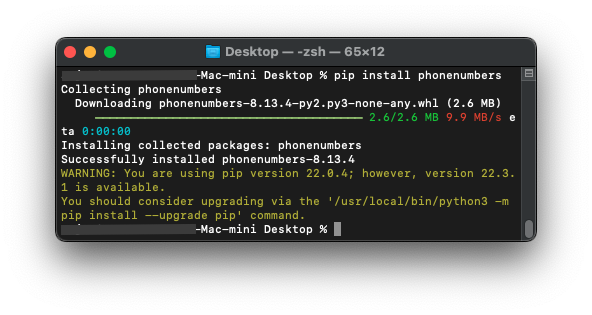
Parsing
You can parse a phone number present in string into a PhoneNumber object using phonenumbers.parse() function.
In the following program, we have a phone number string in variable phonenumber_string
. We parse this string into a PhoneNumber using phonenumbers.parse()
function.
Python Program
import phonenumbers
phonenumber_string = "+442083661177"
x = phonenumbers.parse(phonenumber_string)
print(x)
Output
Country Code: 44 National Number: 2083661177
Validation
phonenumbers.is_valid_number() function performs a full validation of a phone number for a region using length and prefix information.
Python Program
import phonenumbers
#phone number in a string
phonenumber_string = "+442083661177"
#parse string to a PhoneNumber object
x = phonenumbers.parse(phonenumber_string)
#check if the PhoneNumber is a valid number
if phonenumbers.is_valid_number(x):
print('Given phone number is valid.')
else:
print('Given phone number is not valid.')
Output
Given phone number is valid.
phonenumbers.is_possible_number() function quickly guesses whether a number is a possible phone number by using only the length information, much faster than a full validation by phonenumbers.is_valid_number().
Python Program
import phonenumbers
#phone number in a string
phonenumber_string = "+442083661177"
#parse string to a PhoneNumber object
x = phonenumbers.parse(phonenumber_string)
#check if the PhoneNumber is a possible number
if phonenumbers.is_possible_number(x):
print('Given phone number is possible.')
else:
print('Given phone number is not possible.')
Output
Given phone number is possible.
Formatting
phonenumbers.format_number() function formats the given PhoneNumber object to a specified phone number format like NATIONAL, INTERNATIONAL, E164, etc.
Python Program
import phonenumbers
#phone number in a string
phonenumber_string = "+442083661177"
#parse string to a PhoneNumber object
x = phonenumbers.parse(phonenumber_string)
#format PhoneNumber to NATIONAL
formatted = phonenumbers.format_number(x, phonenumbers.PhoneNumberFormat.NATIONAL)
print('NATIONAL : ', formatted)
#format PhoneNumber to INTERNATIONAL
formatted = phonenumbers.format_number(x, phonenumbers.PhoneNumberFormat.INTERNATIONAL)
print('INTERNATIONAL : ', formatted)
#format PhoneNumber to E164
formatted = phonenumbers.format_number(x, phonenumbers.PhoneNumberFormat.E164)
print('E164 : ', formatted)
Output
NATIONAL : 020 8366 1177
INTERNATIONAL : +44 20 8366 1177
E164 : +442083661177
phonenumbers library Tutorials
The following tutorials cover different use cases related to phone numbers.
- Python – Parse phone number
- Python – Validate phone number
- Python – Format phone number
- Python – Get country code from phone number
- Python – Get country name from phone number
Summary
In this tutorial of Python Examples, we learned about phonenumbers library and its functionalities with examples.