Python Try Except - Working Examples
Try Except
try-except is used to handle exceptions thrown the by Python Interpreter at runtime. When the interpreter throws an error, the program execution stops abruptly. To avoid such havoc, we can use try-except statement and handle the exceptions programmatically.
In this tutorial, we shall learn the syntax of try except and how to use try except in Python programs to handle exceptions at runtime.
Syntax
The syntax of try-except statement is as shown in the following.
try:
#your code that may throw exceptions
statement(s)
except Exception1:
#If Exception1 is thrown, then execute this block.
statement(s)
except Exception2:
#If Exception2 is thrown, then execute this block.
statement(s)
else:
#If there is no exception then execute this block.
statement(s)
Include the code that could throw exceptions in try block.
Then except block(s) follow. We can handle multiple exceptions thrown by your code in try block. Suppose if our try block code can throw two types of exceptions, then we can have two except blocks that handle each of these exceptions. We can provide statements for execution separately for each of these Exceptions that could occur using these separate except blocks.
else block is optional. And if we write else block, it is executed only when try block does not throw any exception. In other words, when there is no exception, Python executes else block.
Examples
1. Try-except to handle ZeroDivisionError
In this example, we will try to division of two numbers. If the denominator is zero, an exception is thrown by the Interpreter, and we will catch it in runtime using except block.
Python Program
a = 3
b = 0
c = 0
try:
c = a/b
except ZeroDivisionError:
print('b cannot be zero')
print(c)
Output
b cannot be zero
0
If we do not use try catch as shown in the below program, we will get Exception at runtime. Specifically, ZeroDivisionError for the code.
Python Program
a = 3
b = 0
c = 0
c = a/b
print(c)
Output
Traceback (most recent call last):
File "example.py", line 5, in <module>
c = a/b
ZeroDivisionError: division by zero
2. Multiple Except Blocks
In this example, we will try to typecast given string values to integer, and then divide a number with other. If the string is a not a parsable integer, then we get ValueError. If the denominator is zero, then we get ZeroDivisionError. We shall write two except blocks, one for ValueError, and other for ZeroDivisionError.
Python Program
x = input('Enter numenator : ')
y = input('Enter denomenator : ')
try:
a = int(x)
b = int(y)
c = a/b
except ValueError:
print('Check if input string is parsable integer')
except ZeroDivisionError:
print('Denomenator is zero.')
Output
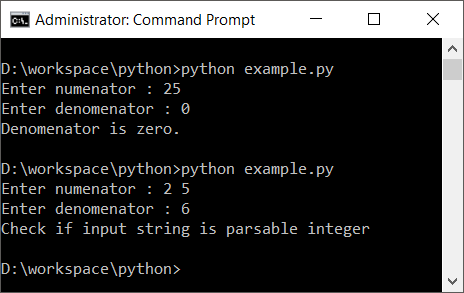
3. Try-Except with Else Block
In the syntax, we have seen that we can write an optional else block after try and except.
In this example, we shall write a try-except with a trailing else block. This else block is executed when no except block catches an error.
We shall take at the same use-case as that of in previous example, but with an else block at the end of try-except.
Python Program
x = input('Enter numenator : ')
y = input('Enter denomenator : ')
try:
a = int(x)
b = int(y)
c = a/b
except ValueError:
print('Check if input string is parsable integer')
except ZeroDivisionError:
print('Denomenator is zero.')
else:
print('No Errors.')
Output
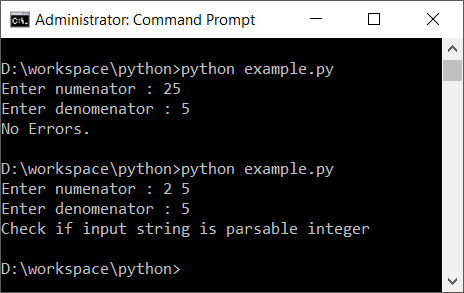
Summary
In this tutorial of Python Examples, we learned how to use try-except statement to handle exceptions in Python programs.