How to Write Comments in Python
Python Comments
Python Comments are used to explain the code and make it more readable to another developer. This is very important for the maintenance of the code, or when you are part of a bigger team.
Python comments are not executed by Python Interpreter. These are the program statements that are ignored.
Most developers find it difficult to understand the code without comments. So, even for personnel projects, writing comments helps you in the long run.
How to write Comments in Python?
A comment in Python starts with #
symbol. Anything that is preceded after #
till the end of that line, is considered a comment.

We can write any text in the comment. Also, we can comment out part of the program, that we would not want to run, but keep it, during initial stages of an application development.
Comments will be not be considered for execution by Python Interpreter. Therefore, comments are only for developer understanding purpose and the comments does not affect program output or behavior.
Single line Comments
1. Example for Single line Comments in Python
The following program contains a comment in the first line.
Python Program
#this is a comment
print('Hello World!')
The first line starting with #
is a single line comment.
Output
Hello World!
The comment is ignored and only the print statement is executed.
2. Comment at the End of Python Statement
We can also write comment after code in that same line. The definition still holds. Anything written after #
in that line is considered comment.
Python Program
print('Hello World!') #this is a comment
Output
Hello World!
3. Commenting Python Code
We can comment code as well, to prevent Python Interpreter from executing this piece of code.
Python Program
#print("Hello World")
print("Welcome to pythonexamples.org")
The first print statement is not executed, since Python interprets the line as a comment.
Output
Welcome to pythonexamples.org
Python Multiline Comments
We can write multiple line comments. There is no another symbol or arrangement to write multi-line comments in Python, but we can use a hack.
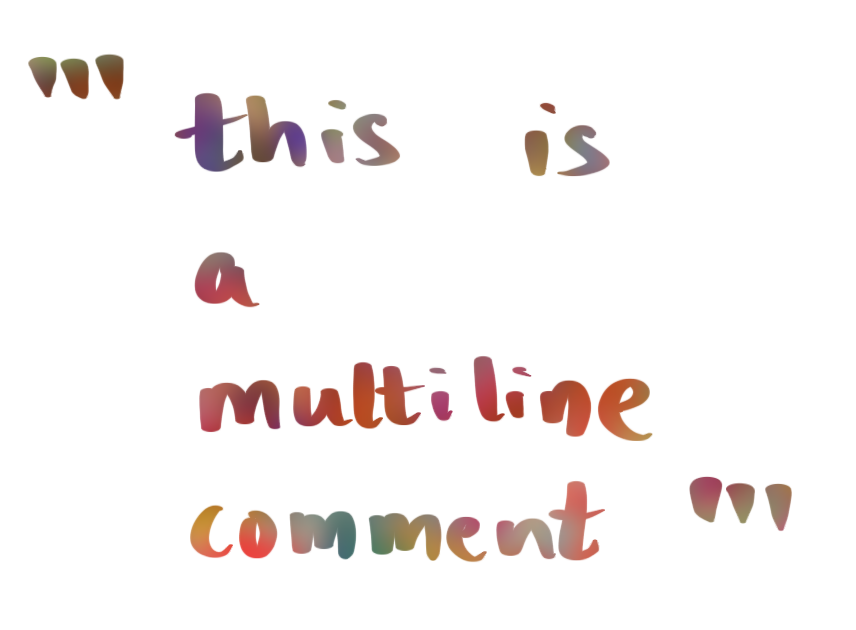
1. Multiline Comments using Multiline String
You can also write a multiple line string, without assigning it to any variable. It does not affect the program output, and therefore can be considered as a comment. An example for multi-line comment using string hack is given below.
Python Program
'''
this is
multi-line string and servers as a comment
'''
print('Hello World!')
Output
Hello World!
2. Multiline Comments using # in Python
You can write multiline comments using #
as shown below.
Python Program
#this is a comment
#this is another comment
#one more comment
print('Hello World!')
Output
Hello World!
Summary
In this tutorial of Python Examples, we have learned how to write single line and multi line comments in Python programming language.