Python BeautifulSoup - Get href of link element
Python BeautifulSoup - Get href of link element
To get the href attribute of a link (<a>) element in Python using BeautifulSoup, you can use Tag.attrs property. The attrs property returns a dictionary with attribute names as keys, and the attribute values as respective values for the keys. You can get the value of href attribute from this dictionary using the 'href'
key.
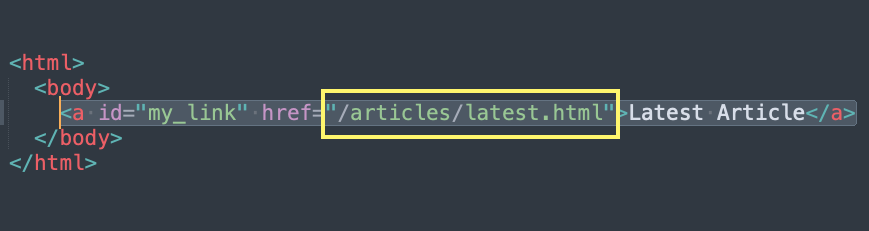
1. Get href attribute of a link element using Tag.attrs property in Python
In the following program, we take a sample HTML content with a link element, get the link element, and then get the value of href attribute for this link element using attrs property.
Python Program
from bs4 import BeautifulSoup
# Sample HTML content
html_content = """
<html>
<body>
<a id="my_link" href="/articles/latest.html">Latest Article</a>
</body>
</html>
"""
# Parse the HTML content
soup = BeautifulSoup(html_content, 'html.parser')
# Consider this as given link element
link_element = soup.find(id="my_link")
# Get element attributes
attributes = link_element.attrs
# Get href attribute
href = attributes['href']
print(href)
Output
/articles/latest.html
The href attribute of the link element <a id="my_link" href="/articles/latest.html">Latest Article</a>
is "
./articles/latest.html"
2. Get href attribute of all link elements in HTML using Tag.attrs property in Python
In the following program, we take a sample HTML content with multiple link elements, get all the link elements, iterate over the link elements, and then get the value of href attribute for each link element using attrs property.
Python Program
from bs4 import BeautifulSoup
# Sample HTML content
html_content = """
<html>
<body>
<a href="/articles/1.html">Article 1</a>
<a href="/articles/2.html">Article 2</a>
<a href="/articles/3.html">Article 3</a>
</body>
</html>
"""
# Parse the HTML content
soup = BeautifulSoup(html_content, 'html.parser')
# Get all link elements
link_elements = soup.find_all("a")
for link_element in link_elements:
# Get element attributes
attributes = link_element.attrs
# Get href attribute
href = attributes['href']
print(href)
Output
/articles/1.html
/articles/2.html
/articles/3.html
Summary
In this Python BeautifulSoup tutorial, given the link element, we have seen how to get the value of href attribute using Tag.attrs property.