Python BeautifulSoup - Get id of HTML Element
Python BeautifulSoup - Get id of HTML Element
To get the id attribute of a HTML element in Python using BeautifulSoup, you can use Tag.attrs property. The attrs property returns a dictionary with attribute names as keys, and the attribute values as respective values for the keys. You can get the value of id attribute from this dictionary.
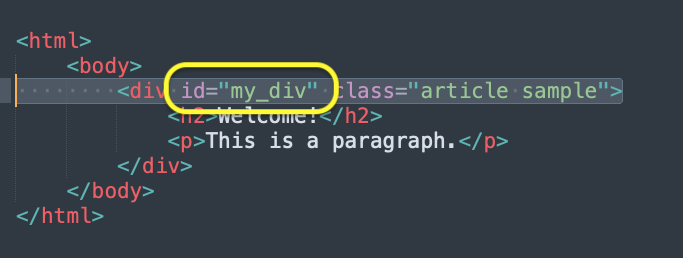
1. Get id attribute of a Div element using attrs property in Python
In the following program, we take a sample HTML content in html_content variable, find the first div element, and then get the id attribute of this div element using attrs property.
Python Program
from bs4 import BeautifulSoup
# Sample HTML content
html_content = """
<html>
<body>
<div id="my_div" class="article sample">
<h2>Welcome!</h2>
<p>This is a paragraph.</p>
</div>
</body>
</html>
"""
# Parse the HTML content
soup = BeautifulSoup(html_content, 'html.parser')
# Consider this as given element
element = soup.find_all("div")[0]
# Get attributes of element
attributes = element.attrs
# Get id from attributes
attr_id = attributes['id']
print(f"id value : {attr_id}")
Output
id value : my_div
The id attribute of the element <div id="my_div" class="article sample">
is my_div
.
Summary
In this Python BeautifulSoup tutorial, given the HTML element, we have seen how to get the id attribute of given HTML element using Tag.attrs property.