Python BeautifulSoup - Get img src
Python BeautifulSoup - Get img src
To get the src attribute of an img (image) element in Python using BeautifulSoup, you can use Tag.attrs property. The attrs property returns a dictionary with attribute names as keys, and the attribute values as respective values for the keys. You can get the value of src attribute from this dictionary using the 'src'
key.
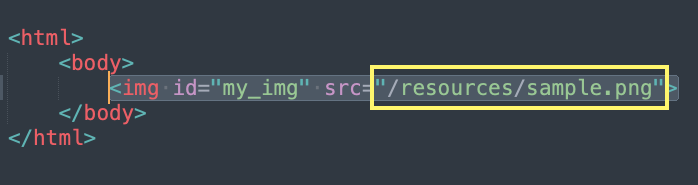
1. Get src attribute of an image element using Tag.attrs property in Python
In the following program, we take a sample HTML content with an image element, get the image element, and then get the value of src attribute for this image element using attrs property.
Python Program
from bs4 import BeautifulSoup
# Sample HTML content
html_content = """
<html>
<body>
<img id="my_img" src="/resources/sample.png">
</body>
</html>
"""
# Parse the HTML content
soup = BeautifulSoup(html_content, 'html.parser')
# Consider this as given image element
img_element = soup.find(id="my_img")
# Get element attributes
attributes = img_element.attrs
# Get src attribute
src = attributes['src']
print(src)
Output
/resources/sample.png
The src attribute of the image element <img id="my_img" src="/resources/sample.png">
is "
./resources/sample.png"
Summary
In this Python BeautifulSoup tutorial, given the image element, we have seen how to get the value of src attribute using Tag.attrs property.