How to Slice a NumPy Array?
NumPy - Array Slicing
Array slicing is a process of reading elements in a specific index range.
If the array is multi-dimensional, we can specify index in multi-dimensions to get the specific array slice.
Slicing 1-D NumPy Array
To slice a 1-D Array from specific starting position upto a specific ending position, use the following syntax.
arr[start:end]
Element at the end
index is not included.
1. Example to slice numpy array with index [1:5]
In the following program, we take a 1-D NumPy Array, and do slicing with the slice [1:5]
.
Python Program
import numpy as np
arr = np.array([1, 2, 3, 4, 5, 6, 7, 8])
print(arr[1:5])
Output
[2 3 4 5]
Explanation
array : [1, 2, 3, 4, 5, 6, 7, 8]
index : 0 1 2 3 4 5 6 7
| |
start end
slice : 2, 3, 4, 5
If start
is not specified, then the starting index of the array 0
is taken. If end
is not specified, then length of the array is taken.
2. Example to slice 1D NumPy Array in steps
We can also slice the array in steps.
The syntax to slice a 1-D NumPy Array in steps is
arr[start:end:step]
In the following program, we take a 1-D NumPy Array, and slice it in steps of 2.
Python Program
import numpy as np
arr = np.array([1, 2, 3, 4, 5, 6, 7, 8])
print(arr[1:5:2])
Output
[2 4]
Explanation
array : [1, 2, 3, 4, 5, 6, 7, 8]
index : 0 1 2 3 4 5 6 7
| |
start end
step=2: * *
slice : 2, 4
Slicing 2-D NumPy Array
Just like a 1-D Array, we can also slice a 2-D Array.
To slice a 2-D Array from specific starting position upto a specific ending position, in the two dimensions, use the following syntax.
arr[start_dim1:end_dim1, start_dim2:end_dim2]
where
start_dim1:end_dim1
is the start and end index of slice in the first dimension.start_dim2:end_dim2
is the start and end index of slice in the second dimension.
1. Example to slice 2D numpy array with index [1, 1:3]
In the following program, we take a 2-D NumPy Array, and slice it.
Python Program
import numpy as np
arr = np.array([[1, 2, 3, 4, 5], [6, 7, 8, 9, 10]])
print(arr[1, 1:3])
Output
[7 8]
Explanation
array : [[1, 2, 3, 4, 5], [6, 7, 8, 9, 10]]
1st dim (indexes) : 0 1
2nd dim (indexes) : 0 1 2 3 4 0 1 2 3 4
arr[1, :] : [6, 7, 8, 9, 10]
arr[1, 1:3] : 7, 8
Slicing [1, 1:3] means get the elements in the second row, from index 1 to 3 (element at index=3 not included).
2. Example to slice 2D numpy array with index [0:2, 1:3]
In the following program, we take a NumPy Array, and slice a 2x2 matrix .
Python Program
import numpy as np
arr = np.array([[1, 2, 3, 4, 5],
[6, 7, 8, 9, 10],
[11, 12, 13, 14, 15],
[16, 17, 18, 19, 20]])
print(arr[0:2, 1:3])
Output
[[2 3]
[7 8]]
Explanation
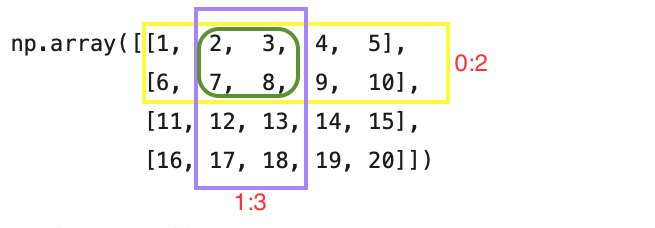
Slicing 3-D NumPy Array
As the number of dimensions increase, just increase the number of slices in the square brackets, separated by comma.
To slice a 3-D Array from specific starting position upto a specific ending position, in different dimensions, use the following syntax.
arr[start_dim1:end_dim1, start_dim2:end_dim2, start_dim3:end_dim3]
where
start_dim1:end_dim1
is the start and end index of slice in the first dimension.start_dim2:end_dim2
is the start and end index of slice in the second dimension.start_dim3:end_dim3
is the start and end index of slice in the third dimension.
Instead of both start:end, we can specify only a single index. In that case, element at that index is only selected for slicing.
In the following program, we take a 3-D NumPy Array, and slice it.
Python Program
import numpy as np
arr = np.array([[[1, 2, 3, 4, 5],
[6, 7, 8, 9, 10]],
[[11, 12, 13, 14, 15],
[16, 17, 18, 19, 20]]])
print(arr[0, 1, 1:4])
Output
[7 8 9]
Summary
In this NumPy Tutorial, we learned how to slice a NumPy Array, how to slice a multi-dimensional NumPy Array, with examples.