Python For Else
Python For Else
In Python, For Else is a construct with a For loop followed by an else block. The else block specifies code that should be executed when the For loop completes normally, without encountering a break statement.
The For Else construct is often used for code that should run if the loop iterates through all its elements without interruption.
Please note that else block does not execute if the For loop encounters a break statement.
In this tutorial, you will learn about for...else construct in Python, its syntax, execution flow, and how to use for...else in programs with the help of examples.
Syntax of For Else
The syntax of For loop with Else block is
for element in iterable:
# Loop body
# ...
else:
# Code to run if the loop completes without a break statement
# ...
Execution flow of For Else
The execution flow of For Else is given below.
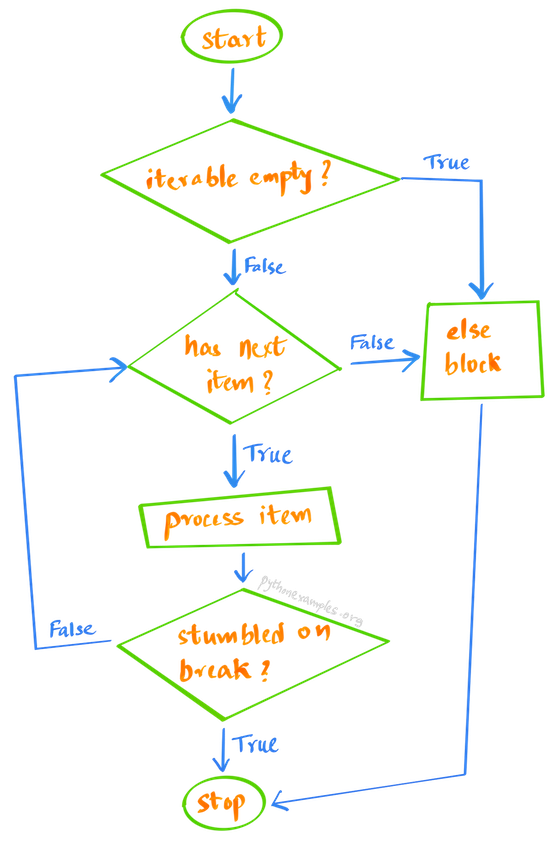
Examples
1. A simple For Else example in Python
In this example, we iterate over a list of strings using a For loop. The For loop has an else block after the For loop.
Python Program
list_1 = ['apple', 'banana', 'cherry']
for item in list_1:
print(item)
else:
print('\nPrinted all the items in iterable.')
Output
apple
banana
cherry
Printed all the items in iterable.
2. For Else with break statement, in Python
The actual use of an Else block with For loop is appreciated when we have to check a specific condition with the items in the iterable.
For example, consider that we have to check if a list of strings list has a string with a length of 3. All we care in this use case is if the given iterable has an item with a length of 3.
How do we do this using a For loop? We take a flag, before the For loop, and inside the for loop, if an item matches the condition that the item length is 3, then we shall set the flag and break the loop, as shown in the following program.
Python Program
list_1 = ['apple', 'cherry', 'mango']
# Flag
is_item_present = False
for item in list_1:
if len(item) == 3:
is_item_present = True
break
if is_item_present:
print('Item with length 3 is found.')
else:
print('Item with length 3 is NOT found.')
Output
Item with length 3 is NOT found.
How can we do this without using a flag? One way for sure is to use For Else.
We know that else in for...else executes if For loop do not encounter a break statement. Using this fact,
- Inside the For loop, we can check the required condition, and if the condition is satisfied, we do the required action, which in this case is just printing out a message that the item is found, and execute a break statement.
- If the condition is not satisfied for any item in the iterable, no break statement is executed, and therefore we have Else block that executes and do the required action.
Let us write the program with the for..else
, not using a flag to check if an item with length 3 is present.
Python Program
list_1 = ['apple', 'cherry', 'mango']
for item in list_1:
if len(item) == 3:
print('Item with length 3 is found.')
break
else:
print('Item with length 3 is NOT found.')
Output
Item with length 3 is NOT found.
This program is more concise and with one less variable, doing the same job, as that of the previous program that uses a flag.
Uses of For Else in Python
The for...else is useful when you want to perform some action after looping through all elements in an iterable without having to introduce additional flags or variables to track whether a specific condition was met within the loop.
Summary
In this tutorial, we learned about for...else in Python, its syntax, execution flow, scenarios where the For loop in for...else has a break statement, and no break statement, and the use case that appreciates the usage of for...else, where we avoided using a flag to check a condition on the items in iterable.