Create Class in Python - Examples
Python - Create Class
To define or create a class in Python, you can use the keyword class
.
Simple example of a class
The syntax of a Python Class starts with the keyword class, followed by class name, and then a semi-colon character :. After an indentation we have the body of class. The body can contain properties and methods.
In the following example, we create a class with the identifier: Person
.
class Person:
name = ""
age = 0
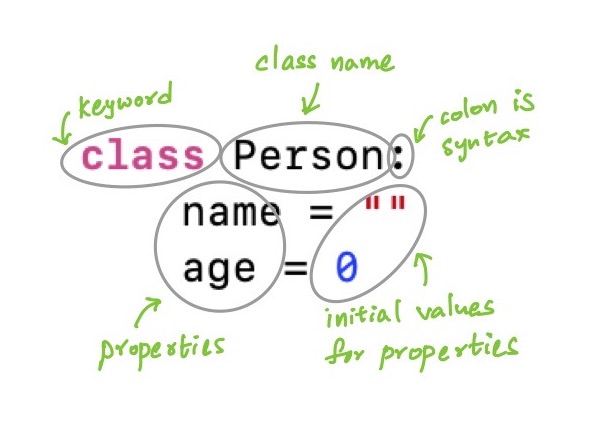
- This class is identified by the literal
Person
. This can also be called as class name or class identifier. - This class has two properties:
name
andage
- The properties has been set with initial (or default) values:
name
with empty string andage
with an integer value of0
.
Create class with methods
Now, let us extend the above example class Person, and add a method named printDetails() to the class. This method call does not take any parameters, however it uses the properties of the class instance.
class Person:
name = ""
age = 0
def printDetails(self):
print("\nPerson Details")
print("Name :", self.name)
print("Age :", self.age)
The self
parameter that is present in the printDetails(self)
is the calling class instance. This is how, we have access to the calling class instance or object.
Summary
In this Python Classes and Objects tutorial, we learned how to write a class with some properties and methods.