Python If NOT
Python If NOT
The logical not operator in Python is used to negate a condition in an If statement. The statements inside the If block execute only if the condition evaluates to False, or if the value (e.g., boolean or collection) is empty.
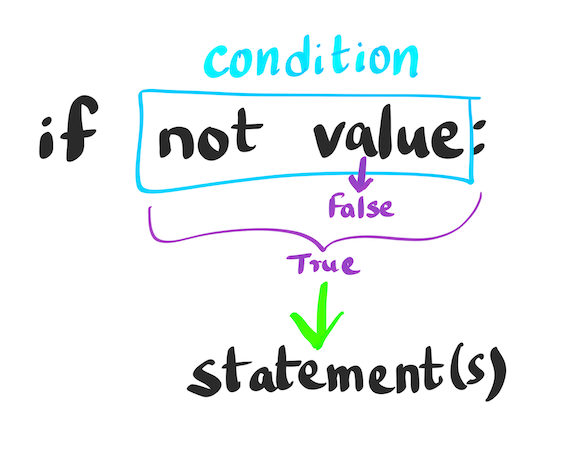
Syntax of If Statement with NOT
The syntax for using the NOT logical operator in a Python If statement is:
if not value:
statement(s)
Here, not value is the condition of the If statement, and value can be of various types, including boolean, string, list, dictionary, set, or tuple.
- For boolean values, not acts as a negation operator. If the value is False, not value will be True, and the code inside the If block will execute. If the value is True, not value will be False, and the code will not execute.
- For strings, lists, dictionaries, sets, and tuples, the condition evaluates to True if the collection is empty.
In summary, we can use the if not expression to conditionally execute a block of code only if the value is empty or False.
Examples
1. Python "if not" with Boolean
In this example, we will use the Python not logical operator in the boolean expression of a Python If statement.
Python Program
a = False
if not a:
print('a is false.')
Since a is False, not a becomes True. As a result, the code inside the If block is executed.
Output
a is false.
2. Python "if not" with String
In this example, we will use Python if not expression to check if a string is empty.
Python Program
string_1 = ''
if not string_1:
print('String is empty.')
else:
print(string_1)
- The variable string_1 is an empty string.
- The boolean equivalent of an empty string is False. Hence, not string_1 becomes True.
- Since the condition is True, the statement inside the If block is executed.
Output
String is empty.
3. Python "if not" with List
In this example, we will use Python if not expression to check if a list is empty.
Python Program
a = []
if not a:
print('Given list is empty.')
else:
print(a)
- The variable a is an empty list.
- The boolean equivalent of an empty list is False, so not a becomes True.
- Since the condition is True, the statement inside the If block is executed.
Output
Given list is empty.
4. Python "if not" with Dictionary
In this example, we will use Python if not expression to check if a dictionary is empty.
Python Program
a = dict()
if not a:
print('Given dictionary is empty.')
else:
print(a)
- The variable a is an empty dictionary.
- The boolean equivalent of an empty dictionary is False, so not a becomes True.
- Since the condition is True, the statement inside the If block is executed.
Output
Given dictionary is empty.
5. Python "if not" with Set
In this example, we will use Python if not expression to check if a set is empty.
Python Program
a = set({})
if not a:
print('Set is empty.')
else:
print(a)
- The variable a is an empty set.
- The boolean equivalent of an empty set is False, so not a becomes True.
- Since the condition is True, the statement inside the If block is executed.
Output
Set is empty.
6. Python "if not" with Tuple
In this example, we will use Python if not expression to check if a tuple is empty.
Python Program
a = tuple()
if not a:
print('Tuple is empty.')
else:
print(a)
- The variable a is an empty tuple.
- The boolean equivalent of an empty tuple is False, so not a becomes True.
- Since the condition is True, the statement inside the If block is executed.
Output
Tuple is empty.
Video Tutorial on Python If not
Watch the following YouTube video for a detailed explanation of the if not statement, including syntax and examples:
Summary
In this tutorial, we learned how to use the NOT logical operator with Python If statements. The if not expression is useful when you need to execute a block of code based on an empty or False value, applied to different data types such as booleans, strings, lists, dictionaries, sets, and tuples.