Contents
Python IF
Python If statement is a conditional statement wherein a set of statements execute based on the result of a condition.
In this tutorial, you’ll learn about Python If statement, its syntax, and different scenarios where Python If statement can be used.
Execution Flow Diagram
Following is a flow diagram of Python if statement. Based on the evaluation of condition, program execution takes one of the paths.
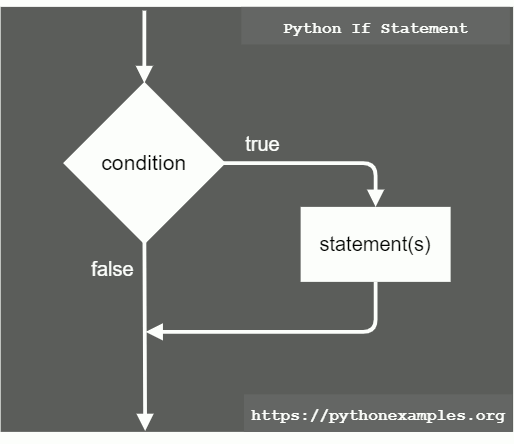
Syntax of If Statement
Following is the syntax of if-statement in Python.
if boolean_expression:
statement(s)
Observe the indentation provided for statement(s) inside if block and the colon : after boolean expression.
If the boolean expression returns true, the statement(s) of the if block are executed. Else, the statement(s) are not executed, and the program execution continues with the statements after if statement, if there are any.
Following is a flowchart depicting the execution of condition and statements in the if statement.
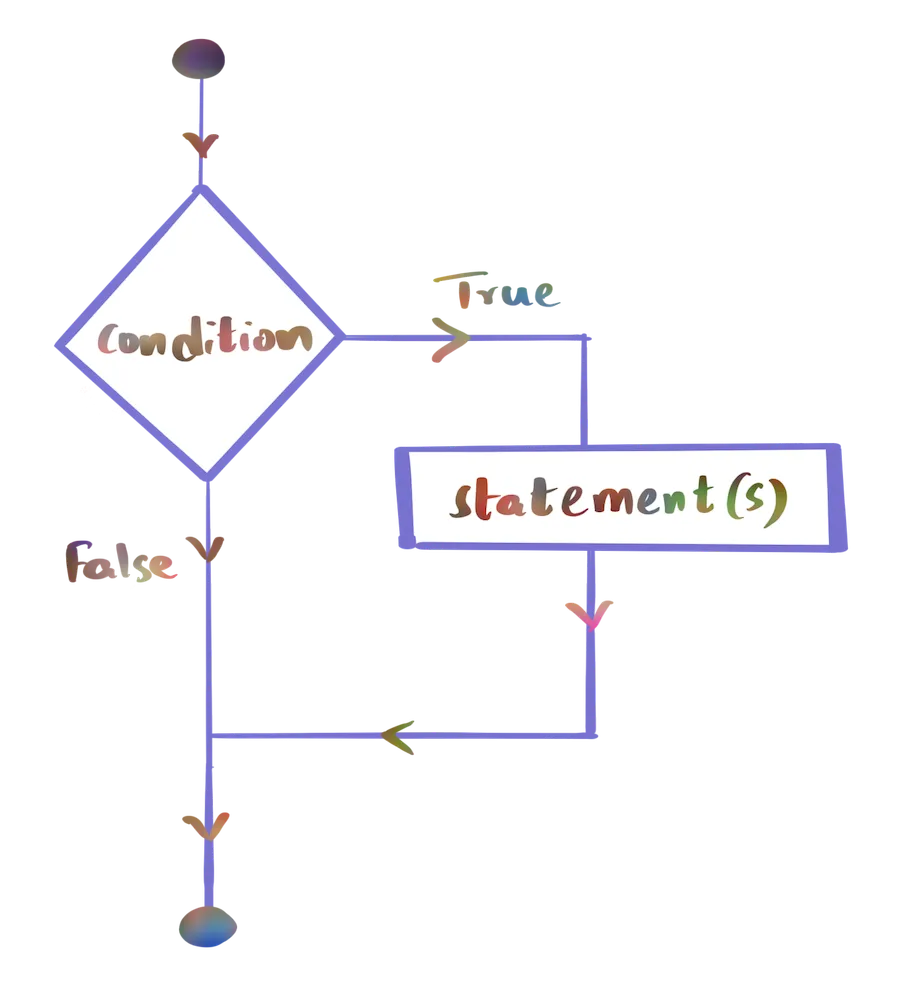
Examples
1. Simple example for If statement
In this example, we will use a simple boolean expression formed with relational operator, less than, for the if statement condition. The statements(s) inside the if block is just a single print statement.
Output
Run the program, and you will see the following output in console.
2 is less than 5
It is trivial that the condition provided in the above if statement evaluates to true, therefore the statement(s) inside the if block is executed.
2. Python If Statement where Boolean Expression is False
In this example, we will write an if statement where the boolean expression or condition evaluates to false.
No Output
The condition provided in the If statement evaluates to False, and therefore the statement inside the if-block is not executed.
3. If statement with compound condition
When you need to join multiple conditions in a single boolean expression, use logical operators to join them and create a compound condition as shown in the following program. The logical operators could be: python and, python or or python not.
Python Program
a = 2
b = 5
c = 4
if a<b and a<c:
print(a, 'is less than', b, 'and', c)
Run Code CopyOutput
2 is less than 5 and 4
a<b is true and a<c is true. As a result the whole condition is True and if-block is executed.
4. If statement with condition evaluating to a number
If the expression in the If statement evaluates to a number, then the statement(s) in if-block are executed if the number is non-zero. zero is considered to be False and non-zero (positive or negative) is considered True.
In this example, we write a Python If statement, where the boolean expression evaluates to a number.
Output
2 is not zero.
As the value of condition evaluates to 2, which is a non-zero number, the statement(s) inside if block are executed.
5. If statement with multiple statements in if-block
In the syntax section, we already mentioned that there can be multiple statements inside if block.
In this example, we write three statements inside if block. Please note that these statements must have same indentation.
Python Program
a = 10
if a > 2 :
print(a, 'is not zero')
print('And this is another statement')
print('Yet another statement')
Run Code CopyWe have written only print statements in this example inside if block. But you may write a set of any valid python statement(s) inside the if block.
Please note that the statements of if-block have same indentation.
Output
2 is not zero
And this is another statement
Yet another statement
6. Nested If statement
You can write a Python If statement inside another Python If statement. This is called nesting.
In this example, we will write a Nested If statement: an If statement inside another If statement.
Python Program
a = 2
if a!=0:
print(a, 'is not zero.')
if a>0:
print(a, 'is positive.')
if a<0:
print(a, 'is negative.')
Run Code CopyOutput
2 is not zero.
2 is positive.
As the first/outer condition a!=0 evaluates to true, the execution enters the if block. And the if statements inside this outer if block are considered as yet another Python statements and executed accordingly.
Summary
In this tutorial of Python Examples, we learned what an If statement is, its syntax, its usage, how to write nested If statement.
Frequently Asked Questions
Answer:
In Python, the if-statement is used for conditional execution of code. It allows you to specify a block of code to be executed only if a certain condition is true.
if condition:
# Code block to execute if condition is True
Answer:
In Python, the if-statement's condition can use comparison equal-to operator ==
to compare two values.
x = 5
y = 5
if x == y:
print('x is equal to y')
Since the values in both x
and y
are equal, the x == y
condition returns true and the if-block executes.
if True
in Python?Answer:
if True
is an if-statement where the condition is always true. Therefore, the if-block always executes.
if True:
#this if-block always executes
print('This code block will always execute')
if False
in Python?Answer:
if False
is an if-statement where the condition is always false. Therefore, the if-block never executes.
if False:
#this if-block never executes
print('This code block will never execute')
Answer:
A nested if-statement in Python is an if-statement that is contained within another if-statement. This means that you have an if-statement inside the code block of another if-statement.
x = 10
y = 5
if x > 5:
print('x is greater than 5')
if y > 2:
print('y is greater than 2')
In this example, there are two if statements. The first if-statement checks if x
is greater than 5. If this condition is true, it executes its code block, which includes another if statement. The nested if statement checks if y
is greater than 2. If both conditions are true, both print statements will execute.