What is a Range in Python
In Python, Range is a sequence of integers within a specified boundaries.
So, how do you define a range
You can define a range using the boundaries. The boundaries are start, and stop. If you would like your range to start at 0, then start would become optional. Also, you can specify a step value, which is the difference between the adjacent values in the range.
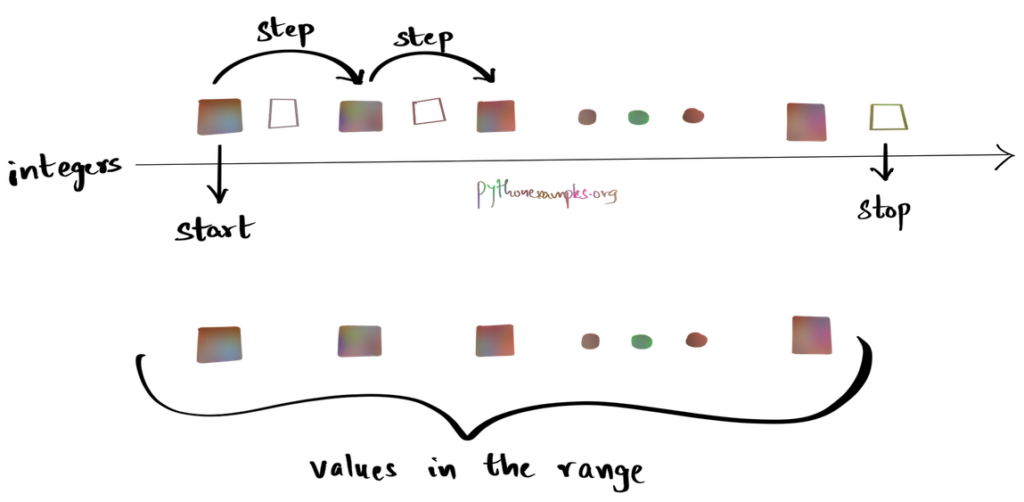
How to Create a Range in Python
To create a range object in Python, you can use the range() built-in function.
The syntax of range() built-in function is
range(stop)
range(start, stop)
range(start, stop, step)
You can use any of the above three definitions to create a range, based on what values you would define a range.
1. Create range with specific stop
range(stop)
range(stop)
creates a range from 0 to stop – 1 with a default step size of 1.
The following is a Python Range with a specific stop value of 9.
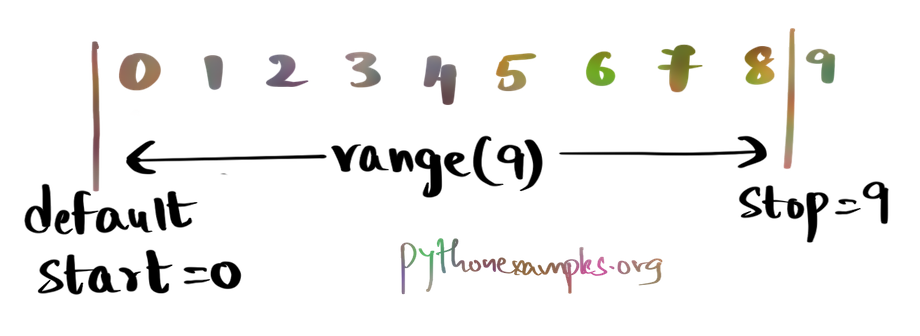
Python Program
# Range with stop value
my_range = range(9)
# Print items in range using list()
print(f"Values in {my_range}\n{list(my_range)}")
Output
Values in range(0, 9)
[0, 1, 2, 3, 4, 5, 6, 7, 8]
2. Create range with specific start and stop
range(start, stop)
range(start, stop)
creates a range from start to stop – 1 with a default step size of 1.
The following is a Python Range with a specific start value of 4 and a stop value of 9.
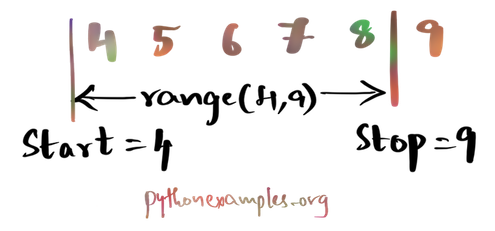
Python Program
# Range with start and stop values
my_range = range(4, 9)
# Print items in range using list()
print(f"Values in {my_range}\n{list(my_range)}")
Output
Values in range(4, 9)
[4, 5, 6, 7, 8]
3. Create range with specific start, stop, and step values
range(start, stop, step)
range(start, stop, step)
creates a range from start to stop – 1 with a custom step size.
The following is a Python Range with a specific start value of 4, stop value of 9, and a step value of 2.
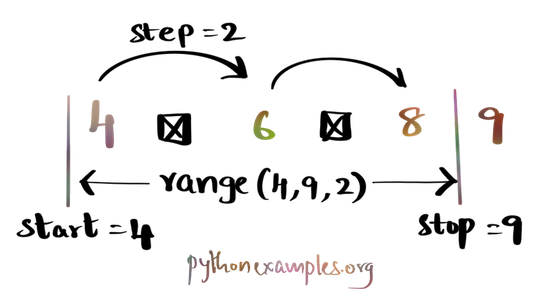
Python Program
# Range with start, stop, and step values
my_range = range(4, 9, 2)
# Print items in range using list()
print(f"Values in {my_range}\n{list(my_range)}")
Output
Values in range(4, 9, 2)
[4, 6, 8]
Negative step value in a Range
We can define a range with a negative step value with start value of the range greater than the stop value.
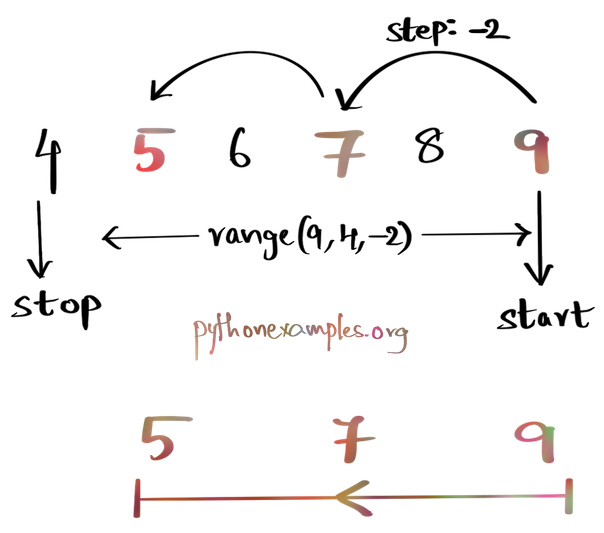
In that case the sequence of numbers in the range would be in descending order.
For example, in the following program, we define a range from 9 to 4 with a step value of -2.
Python Program
my_range = range(9, 4, -2)
print(list(my_range))
Output
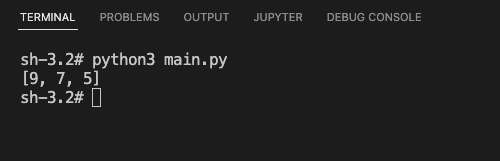
Now, that we know how to define a range in Python, let us see how to print a range to standard output.
Print values in a Range
If you print a range object, it would be just like how we defined it.
For example, consider the following program where we create a range object, and print it to standard output, as is.
Python Program
my_range = range(4, 9)
print(my_range)
Output
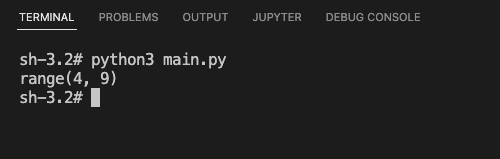
It prints only how we defined the range object, but not the values in the range.
To print the values in the range object, you can use list() built-in function to convert this range into a list, and then print it to output. In that way, you can see all the numbers in the range.
Python Program
my_range = range(4, 9)
print(list(my_range))
Output
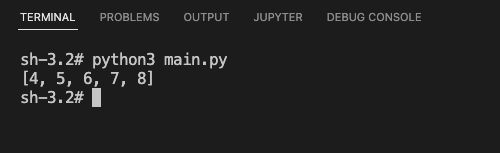
That is how we print the values in a Range. Oh! Good. How do we iterate over the values in a range?
Iterate over a range
You can use a For loop statement to iterate over the values in a given range.
For example, consider the range(4, 9). We shall iterate over the values in this range using a For loop as shown in the following program.
Python Program
my_range = range(4, 9)
for i in my_range:
print(i)
Output
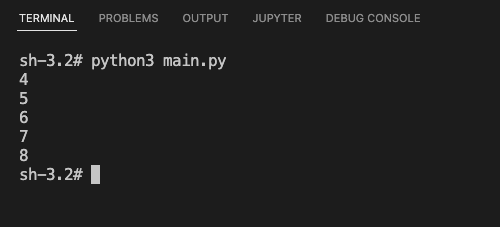
Nice. Now that we have seen how to define a range, how to print a range, and how to iterate over the values in a range, let us dig deep about the range class in Python.
Dig deep into range
Let us have a look into the definition of range class in Python builtins.py.
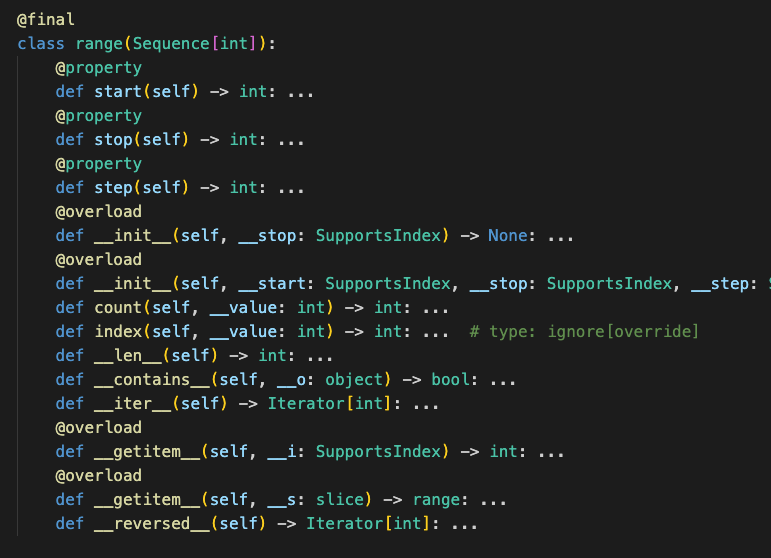
What can we say from this?
- Range is a Sequence of int values.
- range class has three properties: start, stop, and step.
- range class can be initialised with (stop), or (start, stop), or (start, stop, step).
- range class has two methods: count(), and index().
Let us see how we can access the attributes: start, stop, and step; from a range object.
Range object attributes
A range object has three read-only attributes: start, stop, and step. We can access these attributes use dot operator, as shown in the the following program.
Python Program
my_range = range(4, 9, 2)
print(f'Start : {my_range.start}')
print(f'Stop : {my_range.stop}')
print(f'Step : {my_range.step}')
Output
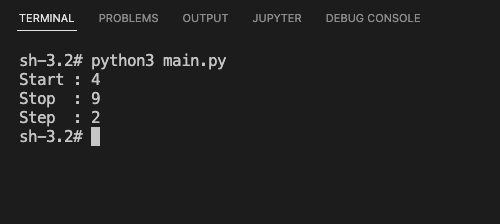
Ranges with Negative Values
In all our previous examples, we have taken ranges of positive numbers only. But, ranges are not limited to positive integers. We can have negative numbers also.
The start value can be negative.
Python Program
my_range = range(-4, 9)
print(list(my_range))
Output
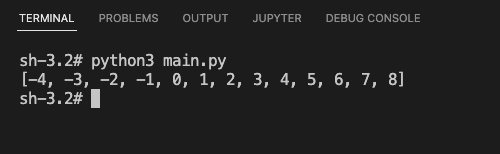
Both the start value and stop value can be negative.
Python Program
my_range = range(-9, -4)
print(list(my_range))
Output
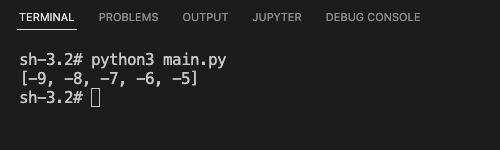
We can have a negative step value also, if the start value of the range is greater than the stop value.
Python Program
my_range = range(-4, -9, -2)
print(list(my_range))
Output
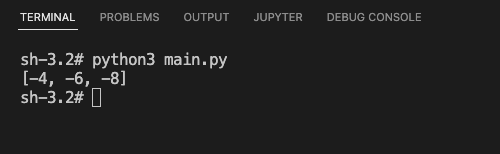
We have got a better idea of what a Range is. Now, let us see if we can convert a range into other types.
Convert range to other datatypes
We have already seen how to convert a range to a list. Similarly, you can use other built-in functions like set(), tuple(), etc., to convert them to the respective datatypes.
Python Program
myrange = range(0, 5)
# Convert range to list
mylist = list(myrange)
print(f'List : {mylist}')
# Convert range to set
myset = set(myrange)
print(f'Set : {myset}')
# Convert range to tuple
mytuple = tuple(myrange)
print(f'Tuple : {mytuple}')
Output
List : [0, 1, 2, 3, 4]
Set : {0, 1, 2, 3, 4}
Tuple : (0, 1, 2, 3, 4)
Range Operations and Manipulations
We can use built-in functions like len(), reversed(), etc., to access the length of a range, reverse a range, respectively.
Also, we can use techniques like slicing to extract subset of a range.
The following tutorials cover all the topics related to Ranges in Python.
Range Basics
- Python range() built-in function
- Python Range attributes
- Python Range length
- Python Range with step
- Python Range with negative step
- Python Range – Print values
- Python – Print range to a single line in output
- Python – Inclusive range
- Python – Negative range
- Python – What does range() function return
- Python – When to use Ranges
Range with loops
- Python – For element in range
- Python – Iterate over range using While loop
- Python – Iterate over alphabets using range()
- Python – Iterate over indices of a list using range()
- Python – Run For loop for specific number of times using range()
- Python – What is range(len())