Inclusive Range in Python
In Python, by default a range is exclusive of the stop value. Meaning, the stop value is not going to be in the sequence generated by the range.
But, if you would like to include the stop value as well in the sequence generated by the range, increment the stop value by one and pass it to the range() function.
range(start, stop + 1)
For example, if you would like to generate a range with the limits [4,9] with 9 in the generated sequence by the range object, pass the stop value as shown in the following.
start = 4
stop = 9
range(start, stop + 1)
Now, the sequence generated by this range would be as shown in the following.
4 5 6 7 8 9
Example
In the following program, we define a range with a start=4, and stop=9. We shall define an inclusive range [4, 9] using range function.
Python Program
start = 4
stop = 9
my_range = range(start, stop + 1)
for i in my_range:
print(i)
Run Code CopyOutput
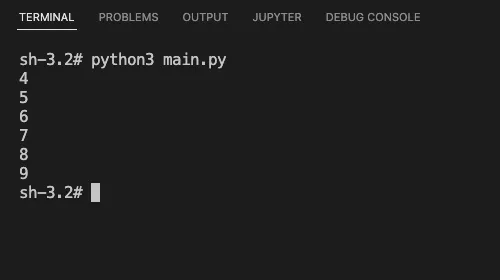
Summary
In this tutorial of Python Ranges, we learned how to generate an inclusive range, that includes the stop value as well in the generated sequence, with examples.