Range Attributes
In Python, a range object has following three attributes or properties.
- start
- stop
- step
The following is a screenshot of the range class definition from Python core file builtins.pyi, with the above mentioned properties.
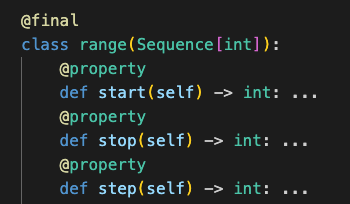
Now, let us have a look at some example programs, where we take a range object and read these attribute values.
Examples
1. Print the properties of a range defined with start and stop
In the following program, we take a range defined by a specific start and stop values. And then we read the three properties and print them to standard output.
Python Program
myrange = range(0, 5)
start = myrange.start
stop = myrange.stop
step = myrange.step
print(f'Start : {start}')
print(f'Stop : {stop}')
print(f'Step : {step}')
Output
Start : 0
Stop : 5
Step : 1
The step value is 1
because if we do not mention a step value when defining a range object, the default step value would be 1
.
2. Print the properties of a range defined with start, stop, and step
In the following program, we take a range defined by a specific start and stop values. And then we read the three properties and print them to standard output.
Python Program
myrange = range(0, 20, 3)
start = myrange.start
stop = myrange.stop
step = myrange.step
print(f'Start : {start}')
print(f'Stop : {stop}')
print(f'Step : {step}')
Output
Start : 0
Stop : 20
Step : 3
Summary
In this tutorial of Python Ranges, we learned how to read the properties/attributes of a range object.