range() Built-in Function -
Python - range()
Python range() builtin function is used to create an immutable sequence of numbers defined by a specific start, end, and a step value.
In this tutorial, you will learn the syntax of range() function, and then its usage with the help of example programs.
Syntax of range() function
range()
function is a constructor of range class. Following is the syntax of range().
range(stop)
range(start, stop[, step])
where
Parameter | Description |
---|---|
start | start represents the starting of the range. start is inclusive in the range. Meaning, the range() returns a range that contains start as first element. If start is not specified, 0 is taken as start. |
stop | stop represents the end of the range. stop is not inclusive in the range. Meaning, the range() returns a range that can be iterated only until stop, but not stop. |
step | step represent the value by which the elements are updated from start to end. If step is not specified, then 1 is taken as default value for step. |
All start, stop and step must be integers.
If you provide only one argument to range()
function, the first form of range() function in the above syntax range(stop)
shall be used with default value of start=0
. Internally range(stop)
calls the constructor range(start, stop)
with start=0
.
If you provide two or three arguments, then the second form of the constructor range(start, stop[, step])
shall be considered.
Examples
1. Range with only stop
When we give only one argument to range(), the argument is considered as stop. The default value of start is 0 and step is 1.
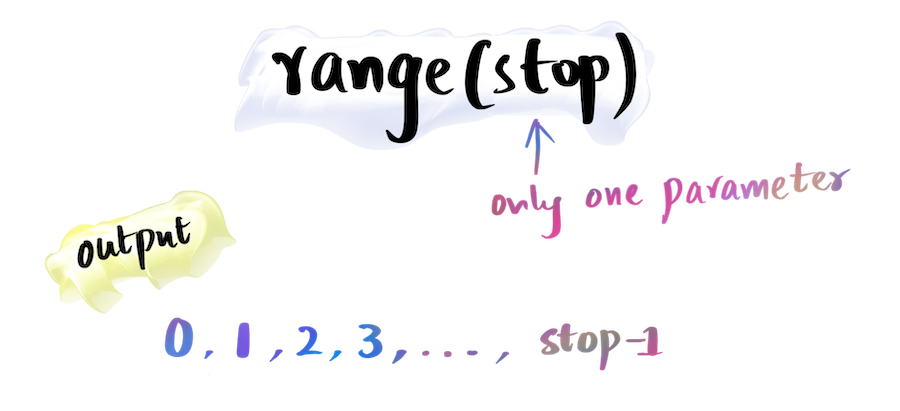
In the following program, we call range() function with only stop parameter.
Python Program
for r in range(5):
print(r)
Run the above program, and the contents of the range(5) are printed to the console one after another in a new line.
Output
0
1
2
3
4
2. Range with start and stop
When you provide two arguments to range(), the first is start and the second is stop. The default value of step is 1, and therefore the contents of range has elements starting from start, in steps of 1 until stop.
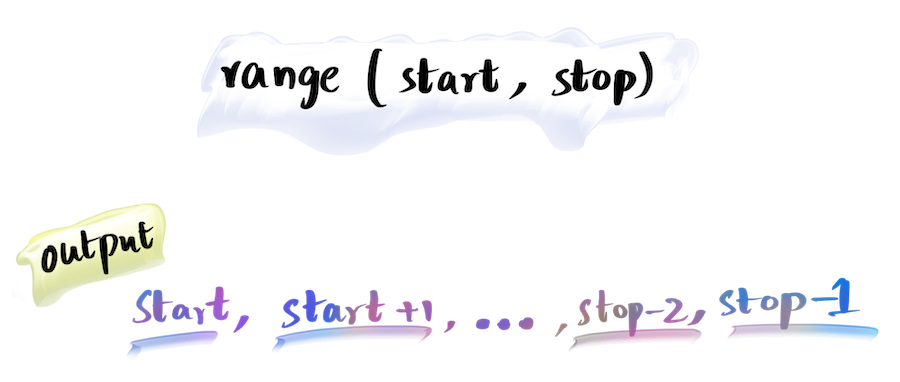
In the following program, we call range() function with specific start and stop parameters.
Python Program
for r in range(4, 9):
print(r)
Run the above program and you should get the range with contents starting at 4 incrementing in steps of 1 until 9.
Output
4
5
6
7
8
3. Python range() with positive step
When you provide a positive step to range(), contents of the range are calculated using the following formula.
r[i] = start + (step * i) such that i>=0, r[i]<stop
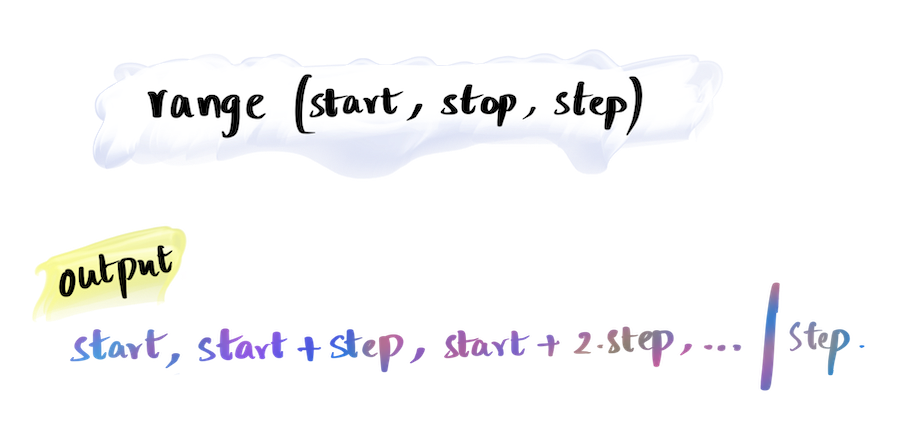
For example, if start=2
, stop=8
and step=2
, then the contents of range are calculated as given below.
r[i] = start + (step * i) < stop
r[0] = 2 + (2 * 0) = 2 < 8 true, this goes into the range
r[1] = 2 + (2 * 1) = 4 < 8 true, this goes into the range
r[2] = 2 + (2 * 2) = 6 < 8 true, this goes into the range
r[2] = 2 + (2 * 3) = 8 < 8 false, this does not go into the range, and the range stops here
In the , we shall iterate over a range, with positive step, and during each iteration, let us print the range element.
Python Program
for r in range(2, 8, 2):
print(r)
Output
2
4
6
4. Range with negative step
When you provide a negative step to range(), contents of the range are calculated using the following formula. The formula remains same as that of with positive step. But the main difference is that the step itself is negative.
r[i] = start + (step * i) such that i>=0, r[i]>stop
Usually, when step is negative, start is greater than the stop, and r[i] should be greater than stop.
For example, if start=8
, stop=2
and step=-2
, then the contents of range are calculated as given below.
r[i] = start + (step * i) < stop
r[0] = 8 + (-2 * 0) = 8 > 2 true, this goes into the range
r[1] = 8 + (-2 * 1) = 6 > 2 true, this goes into the range
r[2] = 8 + (-2 * 2) = 4 > 2 true, this goes into the range
r[2] = 8 + (-2 * 3) = 2 > 2 false, this does not go into the range, and the range stops here
In the following program, we shall iterate over a range, with negative step, and during each iteration, let us print the range element.
Python Program
for r in range(8, 2, -2):
print(r)
Output
8
6
4
5. Access elements of range using index
Just like any sequence, you can access elements of a range using index.
The same formula we used to compute ith element in a range, with a positive or negative step can be used to compute the element using index.
In the following program, we shall define a range, range(2, 100, 8), and print elements at index 5 and 7.
Python Program
r = range(2, 100, 8)
print(r[5]) # r[5] = 2 + [8 * 5]
print(r[7]) # r[7] = 2 + [8 * 7]
Output
42
58
Advantages of range over list or tuple
You may wonder that why we need a separate class for range, while we can do these operations that range does, with list of tuple also. Let us see, why we have a range.
In a list or tuple, their storage in memory is linear with the number of elements. But a range is stored only with values start, stop and end. So, whatever the range of elements is, or how much size the range is, a range is stored in memory only with the three values of start, stop and end.
This makes a huge difference in performance during program execution.
Initialize a list with range
You can initialize a Python List using a range object.
In the following Python Program, we shall initialize a Python List with numbers in the range(4, 9).
Python Program
x = list(range(4, 9))
print(x)
list() constructor can take a range as argument and create a list from these range elements.
Output
[4, 5, 6, 7, 8]
Summary
In this tutorial of Python Examples, we learned the syntax of range(), and how to use range in different scenarios with respect to its arguments, with the help of well detailed example programs.