help() Built-in Function
Python - help()
Python help() built-in function is used to invoke built-in help system for given request. The request could be for objects such as functions, modules, classes, and methods.
If no argument is given to help(), then it starts an interactive help session where you can enter the name of the object you want help with.
In this tutorial, we will learn the syntax and usage of help() built-in function with examples.
Syntax
The syntax of help() function is
help(request)
where
Parameter | Description |
---|---|
request | [Optional] A request such as for a function, module, class, or method. |
Examples
1. help() with no argument
In the following program, we print all the local symbols to standard output.
Python Program
def printCoordinates(x, y):
'''Prints given coordinates.
Parameters:
x (number):X-coordinate
y (number):Y-coordinate
Returns:
None.'''
x = 20
y = 30
print(f'(x, y) = ({x}, {y})')
printCoordinates(20, 30)
help()
Output
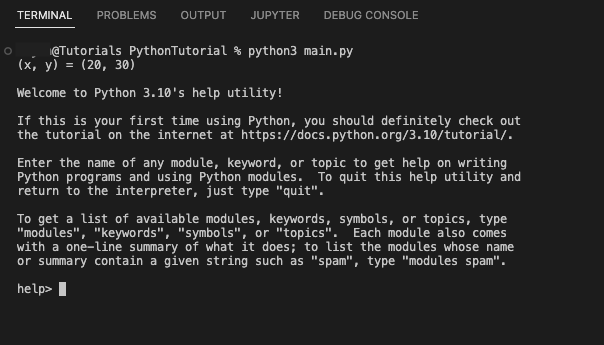
2. help() with function name as argument
In the following program, we define a function printCoordinates()
. Also, we have given a docstring for the function. When we call help() function with the function name passed as argument, the function prints the docstring for the function to the standard output.
Python Program
def printCoordinates(x, y):
'''Prints given coordinates.
Parameters:
x (number):X-coordinate
y (number):Y-coordinate
Returns:
None.'''
x = 20
y = 30
print(f'(x, y) = ({x}, {y})')
printCoordinates(20, 30)
help(printCoordinates)
Output
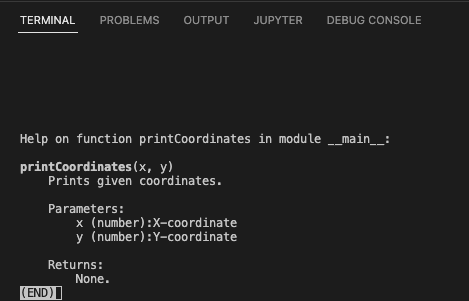
Use cases of help() built-in function
The following are some of the common use cases for the help() built-in function.
- Learning about new objects: If you encounter a new function, module, or class in your code or in documentation, you can use help() to learn more about it. This can help you understand its purpose, usage, and arguments, and can also point you to related functions or modules that you may find useful.
- Debugging: If you are having trouble with a particular function or method, you can use help() to understand its expected behavior and arguments. This can help you identify issues with your code, such as incorrect arguments or unexpected return values.
- Exploring the standard library: The Python standard library contains a wide variety of modules and functions that can be useful for a wide range of applications. You can use help() to explore these modules and functions and discover new ways to solve problems or improve your code.
Summary
In this Built-in Functions tutorial, we learned the syntax of the help() built-in function, and how to use this function to get the built-in help, with examples.