Python print() built-in function - Syntax, Examples
Python print() - Print to Console
Python print() built-in function is used to print given value to console output or a stream.
print() function can take different type of values as argument(s), like string, integer, float, etc., or object of a class type.
The following is a simple demonstration of how to use print() function in a Python shell.
Open a Python shell,
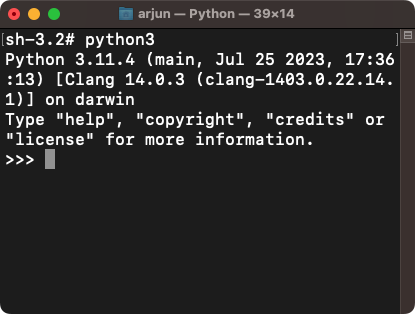
And write the following print statement.
print("Hello World!")
This print statement prints a string of "Hello World!"
to the standard output.
You can also print any other data type. For example let us print a number, say 10
.
print(10)
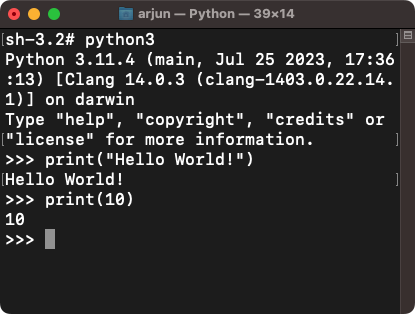
Syntax of print()
The syntax of print() function is
print(*objects, sep=' ', end='\n', file=None, flush=False)
where
Parameter | Description |
---|---|
*objects | Required One or more Python objects. |
sep | Optional A string value. This value is used to join the given objects, and then the resulting string is printed to the output. Default value is single space. |
end | Optional A string value. This value is printed to the output after printing all given objects. Default value is new line. |
file | Optional A Python object with a write(str) method.For example, you can write to file with print() function, using this parameter, since file object has write(str) method. |
flush | Optional A boolean value. Specifies whether the stream has to be flushed. Default value is False. |
As we have already mentioned, you can pass in a string object, number, or any other datatype to print() function as argument, to print to the console.
Also, please note that, you can pass one or more objects or values as arguments to the print() function. print() function can handle all those arguments using separator and end parameters to print all them to the console.
Examples
In the following examples, we shall see how to print values of different datatypes, how to print multiple objects, how to use sep and end parameters of the print() function, etc.
1. Print string value to console output in Python
In this example, we shall print a string to console output. Pass string as argument to the print() function.
Python Program
print('Hello World')
Yeah! That one print statement is all of the program. Run it from your command prompt or terminal using python command. Or you can run it from an IDE like Visual Studio Code.
Output
The string is printed to the console as shown below.
Hello World
Explanation
- The string
'Hello World'
is passed as an argument to theprint()
function. - The function writes the string to the console.
- No additional configuration is required for simple text outputs.
2. Print number to console output in Python
In this example, we will pass integer (number) as argument to print() function and print the number to the console.
Python Program
print(526)
Output
526
Explanation
- The integer
526
is passed as an argument toprint()
. - Python automatically converts the integer to a string before printing.
- The result is displayed as plain text in the console.
3. Print variables to console output in Python
You can provide multiple values to print() function as arguments. They will be printed to console with single space as a default separator. Again, you can specify your own separator, and we shall see that in our next example.
Python Program
x = 'pi is'
y = 3.14
print(x,y)
Output
pi is 3.14
Please note that space is printed out to console, between x
and y
.
Explanation
- Two variables
x
andy
are defined with string and float values, respectively. - The variables are passed as arguments to
print()
. - A single space is used as the default separator between the variables in the output.
4. Print with a specific separator between values in Python
You can pass a string for named parameter sep to override the default separator.
Python Program
x = 'pi is'
y = 3.14
print(x, y, sep=' : ')
Output
pi is : 3.14
Explanation
- The variables
x
andy
are passed toprint()
along with thesep
parameter. - The
sep
parameter specifies the string used to separate the printed values. - The output displays the values separated by the specified string
' : '
.
5. Print with a specific end value in Python
We already know that we can specify an ending value for print() function. New line character is the default ending for print() function in Python. You can override this by passing a string to the named parameter end as shown in the below Python program.
Python Program
print('Hello', end='-')
print('World', end='.\n')
Output
Hello-World.
Explanation
- The
end
parameter specifies what should be printed after the string, replacing the default new line character. - The first
print()
ends with a hyphen ('-'
), and the second ends with a dot and new line ('.\n'
). - The output combines both lines as a single statement based on the specified
end
values.
Video Tutorial for print() in Python
Summary
In this tutorial of Python Examples, we learned how to print a message to console, a variable or any other datatype to the console, using Python print() builtin function with the help of well detailed Python programs.