Python – open()
Python open() built-in function is used to open a file given by specific path, and in specified mode.
In this tutorial, we will learn the syntax and usage of open() built-in function with examples.
Heads up! There are many parameters for this function. But, do not worry. We use only one or two of these in typical programs. And we shall explain these in examples. But for completeness of this builtin function tutorial, we are going to mention all of the parameters, so that you would know what all this open() function can do.
Syntax
The syntax of open() function is
open(file, mode='r', buffering=- 1, encoding=None, errors=None, newline=None, closefd=True, opener=None)
where
Parameter | Optional/ Mandatory | Description |
---|---|---|
file | Mandatory | This is a path-like object giving the pathname to the file. This can be absolute path or relative path to the current working directory. |
mode | Optional | The mode in which the file has to be opened. A separate table for this parameter is given below. |
buffering | Optional | Specifies the buffering policy. |
encoding | Optional | The name of encoding used to encode or decode the file. |
errors | Optional | Specifies how encoding and decoding errors are to be handled. |
newline | Optional | Specifies how newline characters from the file stream are to be handled. |
closefd | Optional | A boolean value specifying a file descriptor for closing the file. |
opener | Optional | A callable function can be passed for this parameter that returns an open file descriptor. |
mode can take any of the following character. The meaning of each character is also given.
Character | Meaning |
---|---|
'r' | Opens file in reading mode. You cannot edit file in this mode. |
'w' | Open file in writing mode. If there is any content in the file, the content is truncated. |
'x' | Open file with the exclusive operation for creating the file. If the file already exists, then open() function fails. |
'a' | Opens file in writing mode. If the file already exists, then it appends the new content to the end of file. |
'b' | Opens file in binary mode, where you can read the bytes of the file. |
't' | Opens the file in text mode. This is the default mode for open() function. |
'+' | Opens file for updating. You can both read from the file, and write to the file. |
Some of these options can be combined, as shown in the following.
mode | Meaning |
---|---|
'w+' or 'w+b' | Opens file in write mode, and truncates the file if there is any content. |
'r+' or 'r+b' | Opens the file, and does not truncate the file if there is any content. |
'rt' | Same as 'r' mode. |
Examples
In the following examples, we will go through programs using open() function to open a file in default mode, write mode, append mode, etc.
1. Open file in default mode (text)
In the following program, we will open a text file given by the relative path data/sample.txt
.
Python Program
filepath = "data/sample.txt"
myFile = open(filepath)
print(myFile)
Output
<_io.TextIOWrapper name='data/sample.txt' mode='r' encoding='UTF-8'>
We just printed out the file object. To display the content of the file, call read() function on the file object.
Python Program
filepath = "data/sample.txt"
myFile = open(filepath)
print(myFile.read())
myFile.close()
Output
Hello World
Yeah ‘Hello World’ is our file content.
2. Open file in write mode, and override content
In the following program, we will open the text file in write mode, and override the content with a string value 'Welcome to new world!'
.
Python Program
filepath = "data/sample.txt"
myFile = open(filepath, 'w')
myFile.write('Welcome to new world!')
myFile.close()
File contents
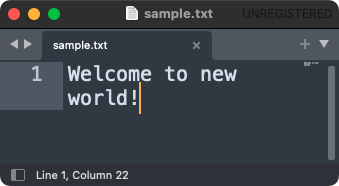
3. Append content to file
In the following program, we will open the text file in append mode, and append the content with a string value ' apple banana cherry'
.
Python Program
filepath = "data/sample.txt"
myFile = open(filepath, 'a')
myFile.write(' apple banana mango')
myFile.close()
File contents
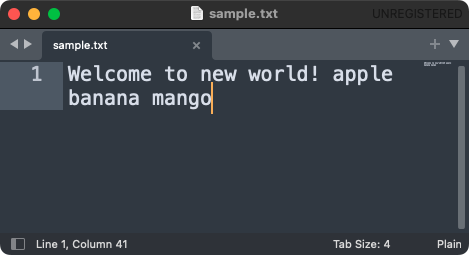
Summary
In this Built-in Functions tutorial, we learned the syntax of the open() built-in function, and how to use this function to open a file in specific mode, and read or modify the file.