Print Range Values to a Single Line in Python
Print Values in a Range to a Single line in Output in Python
To print values in a range to a single line in the standard output in Python, you can iterate over the values in a range using a loop and print them to output using print() statement without new line. Or you can convert the range into a collection like list, or tuple, and then print that collection to output.
In this tutorial, you will learn how to print the values in a range in a single line in the standard output, with examples.
1. Print Range in a Single Line using For Loop
In the following program, we define a range with a start=4, and stop=9. We shall use for value in range statement to iterate over the value of the range, and print them to output using the print() statement without trailing new-line character.
Python Program
my_range = range(4, 9)
for value in my_range:
print(value, end=" ")
print()
Output
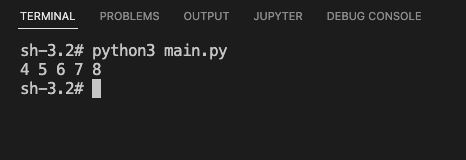
2. Print Range in a Single Line using list()
In the following program, we use Python list() built-in function to convert the given range into a list, and then print the list to output. This way, we can print all the values in the range to a single line.
Python Program
my_range = range(4, 9)
my_list = list(my_range)
print(my_list)
Output
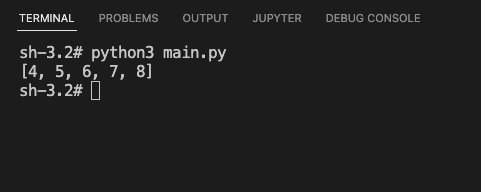
Summary
In this tutorial of Python Ranges, we learned how to print the values of a range object to a single line in the output, with the help of examples.