Iterate Over a Range Using While Loop in Python
Iterate over Range using While loop in Python
To iterate over a range using While loop in Python, initialize a counter i with the start value of the range. While this counter is less than the stop value of the range, run the while loop. Inside the while loop, increment the counter by step value.
For example, if my_range is the given range object, then the code snippet to iterate over the range using Python While loop is
i = my_range.start
while i < my_range.stop:
print(i)
i = i + my_range.step
Example
In the following program, we define a range with a start=4, and stop=9. We shall use for value in range statement to iterate over the value of the range, and print them to output.
Python Program
my_range = range(4, 9)
i = my_range.start
while i < my_range.stop:
print(i)
i = i + my_range.step
Output
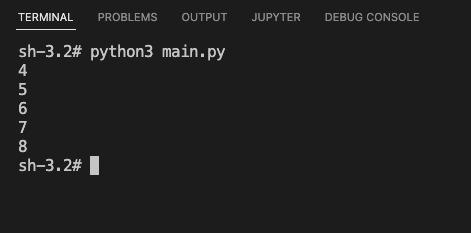
Summary
In this tutorial of Python Ranges, we learned how to iterate over a range using While loop statement, with the syntax and examples.