Contents
Print Values in a Range in Python
If you directly print the range object to output using a print() statement, say print(range(4, 9)), it would be displayed as shown in the following.
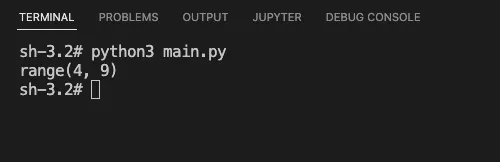
The range is displayed as just how we defined it in the code. If you want to print the values in the range to output, follow this tutorial.
In this tutorial, you will learn how to print the values in a range.
To print values in a range in Python, you can iterate over the values in a range using a loop, and print them to output. Or you can convert the range into a collection like list, or tuple, and then print that collection to output.
1. Print Range using For Loop
In the following program, we define a range with a start=4, and stop=9. We shall use for value in range statement to iterate over the value of the range, and print them to output.
Output
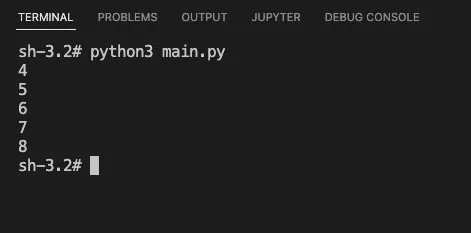
Instead of printing each value in a separate line, we can print all the values of the range in a single line, by printing without trailing new line.
Python Program
my_range = range(4, 9)
for value in my_range:
print(value, end=" ")
print()
Run Code CopyOutput
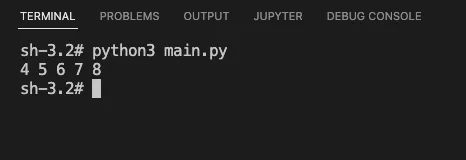
2. Print Range using list()
In the following program, we use Python list() built-in function to convert the given range into a list, and then print the list to output.
Output
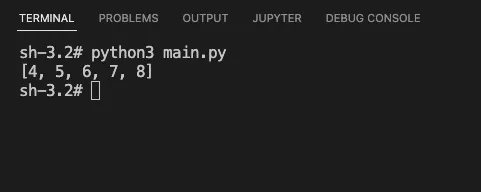
Summary
In this tutorial of Python Ranges, we learned how to print the values of a range object, using For loop statement or by converting the range into a list.