Run For loop for specific number of times using range() in Python
Python Ranges are used to generate a sequence of numbers.
If we can define a range such that it has n number of elements, where n is the number of iteration that we would like to run, then we can use this range object with For loop, and run this For loop for n times.
The syntax to define a range with n number of values is
range(n)
The above function call returns a range object whose start is 0, stop is n, and step value is 1. In other words, it contains the following sequence of integers.
0, 1, 2, 3, ..., n-1
To run a For loop for n times, in Python, you can use range(n)
for the iterator in the For loop syntax as shown below.
for i in range(n):
// your code
Now, let us go through some examples.
1. Repeat For loop for 5 times
In the following program, we define a range function that has five values from 0 to 4, and use it with a For loop to iterate the loop for five times.
Python Program
for i in range(5):
print('Hello World')
Output
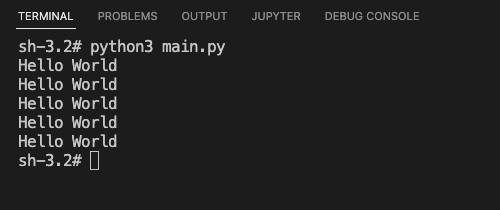
2. Repeat For loop for 10 times
In the following program, we define a range function that has ten values from 0 to 9, and use it with a For loop to iterate the loop for ten times. We shall print the counter (or the current value of the range) inside the loop.
Python Program
for i in range(10):
print(i)
Output
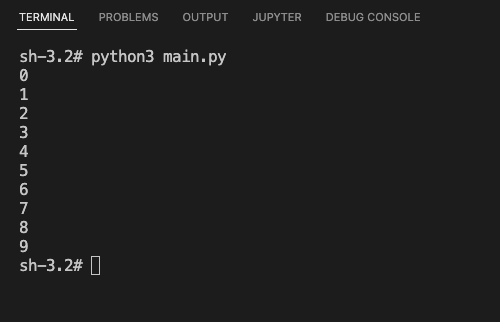
Summary
In this tutorial of Python Ranges, we learned how to run a For loop for specific number of times using range() function.