Iterate Over Alphabets Using Range in Python
Iterate over Alphabets using Range in Python
To iterate over the alphabets using Range in Python,
Create a range object with the unicode point of 'a' as start, and the unicode point of 'z' + 1 as the stop.
start_ch = 'a'
end_ch = 'z'
start = ord(start_ch)
stop = ord(end_ch) + 1
my_range = range(start, stop)
We have initialised our range object. Now, use a For loop statement to iterate over the values in the range. Each value is a unicode point. You may convert this unicode to single character string using chr() built-in function.
for ch in my_range:
print(chr(ch))
You may use ord() built-in function to convert the character to a unicode point.
If you want to iterate over the capital letter alphabets, then take unicode points of 'A' and 'Z' + 1 as start and stop respectively.
start_ch = 'A'
end_ch = 'Z'
Examples
1. Iterate over lowercase alphabets using Range
In the following program, we create a range object with the unicode values of all the lowercase English alphabets, iterate over these values, and print their respective characters to standard output.
Python Program
start_ch = 'a'
end_ch = 'z'
start = ord(start_ch)
stop = ord(end_ch) + 1
my_range = range(start, stop)
for ch in my_range:
print(chr(ch))
Output
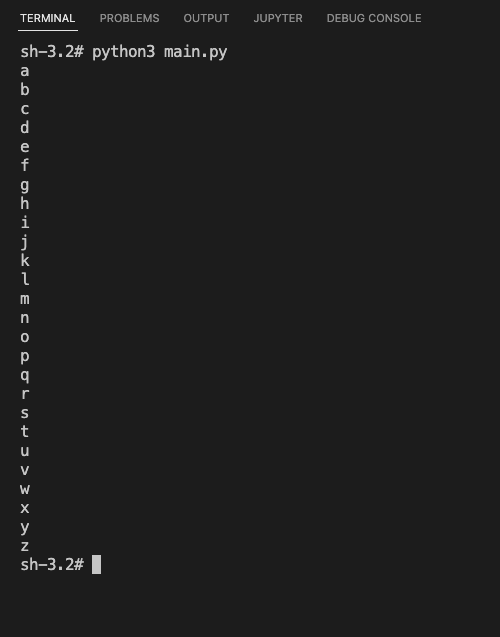
2. Iterate over uppercase alphabets using Range
In the following program, we iterate over the uppercase English alphabets using Range, and print the alphabets to standard output.
Python Program
start_ch = 'A'
end_ch = 'Z'
start = ord(start_ch)
stop = ord(end_ch) + 1
my_range = range(start, stop)
for ch in my_range:
print(chr(ch))
Output
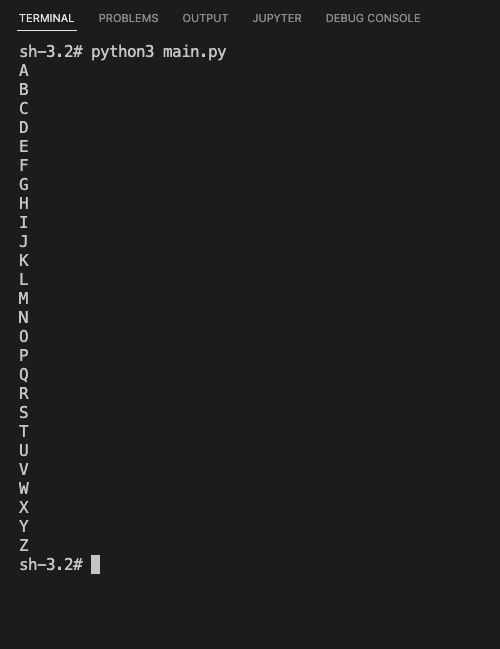
Summary
In this tutorial of Python Ranges, we learned how to iterate over the alphabets using a range, with examples.