Contents
Python Indentation
In Python programming, indentation is the space provided at the beginning of each line of code.
Unlike many other programming languages, where flower braces {} or other mechanism is used to define scope or a block of code, Python does not have those. Instead, it uses indentation.
You can use any number of spaces for indentation. But, you have to provide same number of spaces for statement in a code block.
For example, in the following code snippet, the two print() statements inside a function, have a same number of spaces as indentation.
def myFunction():
print('hello')
print('hello world')
If you provide different number of spaces for the statements inside a block, you will get an error.
In the following program, we write a Python function, with two print statements in it. For the first statement, we will give two spaces, and for the second statement we will give three spaces.
Output
File "d:/workspace/python/example.py", line 3
print('hello world')
^
IndentationError: unexpected indent
At the first statement, you notified Python interpreter that you will use two spaces for the code block that constitute the body of this function. But in the second statement, it found a different indentation, and thrown an error.
Number of Spaces for Indentation
As already mentioned, you can use any number of spaces for indentation, but atleast one space. The number of spaces has to be uniform for a given block.
Python Program
def myFunction():
print('hello')
print('hello world')
def myOtherFunction():
print(1)
print(2)
Run Code CopyIn general Python community uses four spaces to provide indentation.
How to indent a code block inside another?
Writing an if statement requires body (code block) with indentation. Similarly for a looping statement, or a function. We have already seen how to give indentation in such scenarios. But, how to indent set of statements when you have a nested if statements.
In the following program, we will write a nested if statement, and observe how the indentation is provided.
Let us see how this code appears in an editor like Visual Studio Code.
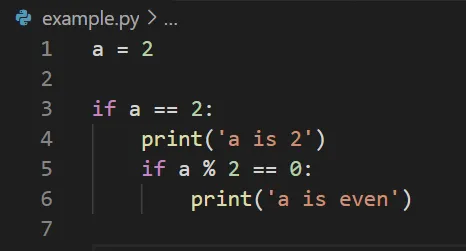
In the above program, line 1 and 3 have same indentation of zero spaces. So, there are only two statements in our Python program.
Now, if you come to if statement in line 3, this if statement has a body containing two statements. You can say this by indentation. Line 4 and 5 are the statements inside this if statement. They have an indentation of 4 spaces.
For the if statement in line 5, there is only one statement with 8 spaces as indentation.
So, for a block inside a block, there has to more number of spaces as indentation, than that of its parent block.
Summary
In this tutorial of Python Examples, we learned about indentation in Python programs, and different aspects of indentation.
Frequently Asked Questions
Answer:
Indentation is the spaces that we provide at the beginning of each line in the code. Indentation is required to specify blocks of code in the program.
Answer:
You can use any number of spaces for indentation in Python. The minimum required is one white space character. Typically, four spaces are used for indentation.