Contents
Python If NOT
We can use logical not operator with Python IF condition. The statements inside if block execute only if the value(boolean) is False or if the value(collection) is empty.
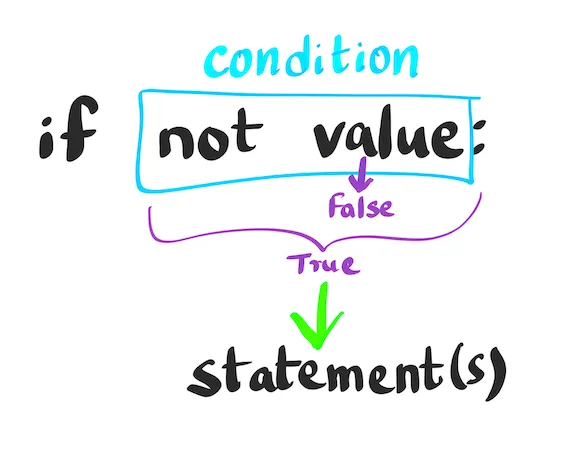
Syntax of if statement with not
The syntax of Python If statement with NOT logical operator is
if not value:
statement(s)
where not value part is the condition of the if statement, and the value could be of type boolean, string, list, dict, set, tuple, etc.
If value is of boolean type, then NOT acts a negation operator. If value is False, not value would be True, and the statement(s) in if-block will execute. If value is True, not value would be False, and the statement(s) in if-block will not execute.
If value is of type string, then statement(s) in if-block will execute if string is empty.
If value is of type list, then statement(s) in if-block will execute if list is empty. The same explanation holds for value of other collection datatypes: dict, set and tuple.
In summary, we can use if not expression to conditionally execute a block of code only if the value is empty or False.
Examples
1. Python “if not” with Boolean
In this example, we will use Python not logical operator in the boolean expression of Python IF statement.
Since a is False, not a becomes True. And as the condition of if statement becomes True, the statements inside the if-block execute.
Output
a is false.
2. Python “if not” with String
In this example, we will use Python if not expression to print the string only if the string is not empty.
Python Program
string_1 = ''
if not string_1:
print('String is empty.')
else:
print(string_1)
Run Code Copy- string_1 is empty.
- The boolean equivalent of an empty string is False. And not string_1 becomes True.
- The condition of if-statement is True. Therefore, the statements inside the if-block execute.
Output
String is empty.
3. Python “if not” with List
In this example, we will use Python if not expression to print the list only if the list is not empty.
- The variable a is assigned with an empty list.
- The boolean equivalent of an empty list is False. And not a becomes True.
- The condition of if-statement is True. Therefore, the statements inside the if-block execute.
Output
Given list is empty.
4. Python “if not” with Dictionary
In this example, we will use Python if not expression to print the dictionary only if the dictionary is not empty.
Python Program
a = dict()
if not a:
print('Given dictionary is empty.')
else:
print(a)
Run Code Copy- The variable a is assigned with an empty dictionary.
- The boolean equivalent of an empty list is False. And not a becomes True.
- The condition of if-statement is True. Therefore, the statements inside the if-block execute.
Output
Given dictionary is empty.
5. Python “if not” with Set
In this example, we will use Python if not expression to print the set, only if the set is not empty.
- The variable a is assigned with an empty set.
- The boolean equivalent of an empty set is False. And not a becomes True.
- The condition of if-statement is True. Therefore, the statements inside the if-block execute.
Output
Set is empty.
6. Python “if not” with Tuple
In this example, we will use Python if not expression to print the tuple, only if the tuple is not empty.
- The variable a is assigned with an empty tuple.
- The boolean equivalent of an empty list is False. And not a becomes True.
- The condition of if-statement is True. Therefore, the statements inside the if-block execute.
Output
Tuple is empty.
Video Tutorial on Python If not
In the following YouTube video, the explanation for if not statement with syntax, and examples is given.
Summary
In this tutorial of Python Examples, we learned to use NOT logical operator along with if conditional statement, with the help of well detailed examples programs.
Frequently Asked Questions
Answer:
Yes, you can use 'if not' in Python. In an if-statement, if you use not operator before the expression, the expression becomes negative, meaning, not expression
is True if the expression is False, and vice versa. We can use 'if not' when we want to execute if-block when the expression in the condition is False.
Answer:
not
operator after the if
keyword modifies the condition with an inverted gate behaviour.
Answer:
The expression not list
is True if the list is not empty, or False if the list is empty. Therefore, when we use this expression with if-statement, the if block executes when the list is not empty.
my_list = [10, 20]
if not my_list:
print('list is not empty')
Answer:
In Python, you use the not
operator with an if-statement to check if a condition is false. It negates the boolean value of the condition. This is useful when you want to execute a block of code if a certain condition is not met.
x = 5
if not x == 10:
print('x is not equal to 10')
In this example, the not operator is used to negate the condition x == 10
. So, the print statement will be executed because the condition x == 10
is false (x
is 5, not 10).