Python – Tuples inside List
List is a collection of items. And tuple can be considered as an item. So, you can have a tuples inside a list in Python.
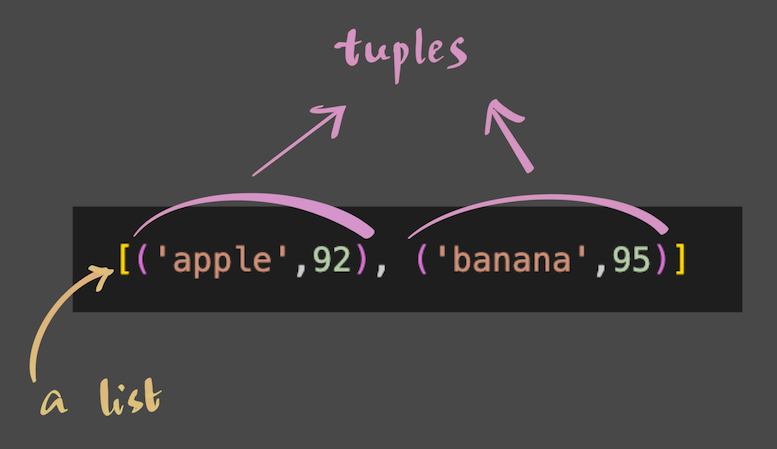
In this tutorial, we will learn how to create tuples inside a list, how to access the tuples in list, how to append a tuple to the list, how to update or remove tuples inside the list, etc., with examples.
Initialize a Tuples inside a List in Python
The following is a simple example to initialize a tuples inside a list. We have a list enclosed in square brackets, where each element is a tuple.
In the above program, we created a list with three tuples inside the list.
Read tuples inside the list in Python
We can read individual tuples inside the list using index notation.
In the following program, we print the tuples present inside the list, one by one, using index.
tuples_in_list = [('apple',92), ('banana',95), ('cherry',93)]
print(tuples_in_list[0])
print(tuples_in_list[1])
print(tuples_in_list[2])
Run Code CopyIf you would like to traverse through the tuples inside the list, then you can use a For loop, and access them in a loop.
tuples_in_list = [('apple',92), ('banana',95), ('cherry',93)]
for tuple_i in tuples_in_list:
print(tuple_i)
Run Code CopyAppend a tuple to the tuples inside list
You can use list append() method to append an tuple to the list.
In the following program, we take a list with three tuples inside it in a variable tuples_in_list, then used list append() method to append a tuple to the list, and the printed all the tuples inside the list in a Python For loop.
Python Program
# Tuples inside a list
tuples_in_list = [('apple',92), ('banana',95), ('cherry',93)]
# Append a tuple to the list
tuples_in_list.append(('fig', 44))
for tuple_i in tuples_in_list:
print(tuple_i)
Run Code CopyOutput
('apple', 92)
('banana', 95)
('cherry', 93)
('fig', 44)
Replace a tuple in the list of tuples
You can modify the items in a list. Therefore, you can replace a tuple in the list with a new tuple.
In the following program, we take a list with three tuples inside it, and then replaced the second tuple with a new one.
Python Program
# Tuples inside a list
tuples_in_list = [('apple',92), ('banana',95), ('cherry',93)]
# Replace a tuple in the list
tuples_in_list[1] = ('fig', 44)
for tuple_i in tuples_in_list:
print(tuple_i)
Run Code CopyOutput
('apple', 92)
('fig', 44)
('cherry', 93)
The second tuple has been replaced in the list, with the given tuple.
Remove a tuple from the tuples inside list
To remove a tuple from the tuples inside the list by index, you can use list pop() method. Call the pop() method on the list, and pass the index of the tuple you would like to remove from the list.
In the following program, we take a list with three tuples inside it, and then removed the second tuple whose index is 1, using pop() method.
Python Program
# Tuples inside a list
tuples_in_list = [('apple',92), ('banana',95), ('cherry',93)]
# Remove a tuple from the list by index
tuples_in_list.pop(1)
for tuple_i in tuples_in_list:
print(tuple_i)
Run Code CopyOutput
('apple', 92)
('cherry', 93)
Summary
In this tutorial, we learned about writing tuples inside a list, how to access the tuples inside a list, how to replace a tuple with a new tuple in the list, how to remove a tuple from the tuples inside a list of tuples, with the help of well detailed Python example programs.