Python - Unpack Tuple
Python - Unpack Tuple
In this tutorial, you will learn what unpacking a tuple means, and how to unpack tuple into variables in Python, with examples.
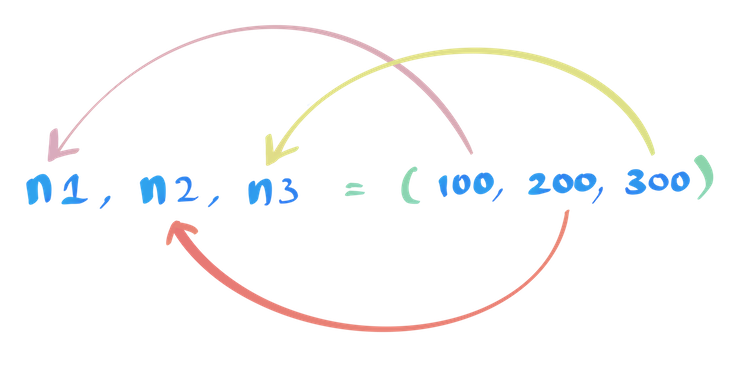
What does unpacking a tuple means?
Let us first look into the situation where we need to unpack a tuple.
Consider a tuple that has three items as shown below.
nums = (100, 200, 300)
If we want to access the items of this tuple, we can simply use index.
For example, to access the first item we can use nums[0]
, and to access the second item we can use nums[1]
, and so on.
But, what if want to store each of these values into individual variables.
We can use index notation, and assign each of the values in the tuple to a separate variable, it would look something like this.
num_1 = nums[0]
num_2 = nums[1]
num_3 = nums[2]
What if I say that there is another way, a more concise way, of doing all these assignments of tuple items to variables within a single statement.
Yes, there is a technique called unpacking.
In the following statement, we shall unpack the tuple items to individual variables.
num_1, num_2, num_3 = nums
Yes! That's it. The items in the tuple nums are assigned to the variables in the respective order. Let us write a program, and verify the same.
Python Program
nums = (100, 200, 300)
# Tuple unpacking
num_1, num_2, num_3 = nums
print(f"num_1 is {num_1}")
print(f"num_2 is {num_2}")
print(f"num_3 is {num_3}")
Output
num_1 is 100
num_2 is 200
num_3 is 300
Also, please note that we have to specify as many variables as that of items in the tuple. For example, in our tuple, there are three items. Therefore, we have to use three variables while unpacking the tuple.
Errors while unpacking a tuple in Python, and how to handle them
If we do not specify as many number of variables as that of values in the tuple, we get ValueError exception. This can occur in two ways.
- When the number of variables is less than the length of given tuple.
- When the number of variables is greater than the length of given tuple.
1. ValueError: too many values to unpack
If we use lesser number of variables while unpacking a tuple, we would get ValueError exception as shown in the following.
In the following program, we tried unpacking a tuple of three items into two variables.
Python Program
nums = (100, 200, 300)
# Tuple unpacking
num_1, num_2 = nums
print(f"num_1 is {num_1}")
print(f"num_2 is {num_2}")
Output
Traceback (most recent call last):
File "/Users/pythonexamplesorg/main.py", line 4, in <module>
num_1, num_2 = nums
^^^^^^^^^^^^
ValueError: too many values to unpack (expected 2)
We get ValueError exception. And the message too many values to unpack is clear, that there are too many values in the tuple to unpack into the expected 2 variables.
Solution
If you are interested in unpacking only the first two items in the tuple to two variables, you have to first unpack the starting required number of items into variables, and then assign the rest into a new tuple using asterisk symbol as shown in the following statement.
num_1, num_2, *others = nums
Let us use this statement to unpack the tuple into fewer number of variables, and run the program.
Python Program
nums = (100, 200, 300)
# Tuple unpacking
num_1, num_2, *others = nums
print(f"num_1 is {num_1}")
print(f"num_2 is {num_2}")
Output
num_1 is 100
num_2 is 200
2. ValueError: not enough values to unpack
If we try to unpack the tuple into more number of variables than the number of items in the tuple, we get ValueError exception. Here is an example, where we try to unpack our tuple of three items into four variables.
Python Program
nums = (100, 200, 300)
# Tuple unpacking
num_1, num_2, num_3, num_4 = nums
print(f"num_1 is {num_1}")
print(f"num_2 is {num_2}")
Output
Traceback (most recent call last):
File "/Users/pythonexamplesorg/main.py", line 4, in <module>
num_1, num_2, num_3, num_4 = nums
^^^^^^^^^^^^^^^^^^^^^^^^^^
ValueError: not enough values to unpack (expected 4, got 3)
And here as well, the message not enough values to unpack is clear that there are not enough values in the tuple to unpack into given number of variables. While the tuple got 3 items, unpacking is expecting 4, because we have given four variables.
Solution
To rectify this issue, you have to make sure that there are as many number of items in the tuple as there are variables.
Summary
In this tutorial of Python Tuples, we have seen what unpacking a tuple means, how to unpack a tuple into variables, different exceptions that could occur while unpacking a tuple and how to handle those exceptions, with example programs.