Contents
Python – Write Dictionary to File
You can write the contents of a dictionary to a file in many ways. You can write the dictionary just as a plain text file, as a JSON file, or as a CSV file.
In this tutorial, you will learn how to write a dictionary to a file as plain text file, as a JSON file, or as a CSV file with example programs.
1. Writing dictionary to a plain text file in Python
We are given a dictionary in my_dict variable with two key-value pairs. We have to write this dictionary to a plain text file.
- Open a file data.txt in write mode using open() built-in function
- Write the dictionary to the file using write() method of file instance. Since write() method takes a string value, we shall convert the dictionary to a string using str() built-in function.
Python Program
my_dict = {
'foo': 12,
'bar': 14
}
with open('data.txt', 'w') as text_file:
text_file.write(str(my_dict))
CopyOutput
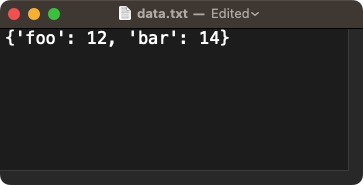
2. Writing dictionary to a JSON file in Python
You can write a given dictionary to a JSON file as a JSON string using json module in Python.
In the following program, we are given a dictionary in my_dict variable with two key-value pairs. We have to write this dictionary to a JSON file.
- Open a file data.json in write mode using open() function.
- Call json.dump() function and pass the dictionary and file object as arguments. dump() method writes the dictionary as a JSON object to the file.
Python Program
import json
my_dict = {
'foo': 12,
'bar': 14
}
with open('data.json', 'w') as json_file:
json.dump(my_dict, json_file)
CopyOutput
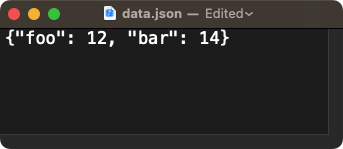
3. Writing dictionary to a CSV file in Python
You can write a given dictionary to a CSV file using csv module in Python. The keys of dictionary becomes the column names or field names in the CSV file, and the dictionary values becomes the rows in the CSV file.
In the following program, we are given a dictionary in my_dict variable with two key-value pairs. We have to write this dictionary to a CSV file.
- Open a file data.csv in write mode using open() function.
- Call csv.DictWriter() function and pass the file object and dictionary keys as arguments to create a CSV writer object.
- Call writeheader() on the writer object to write the header (keys as field names).
- Call writerow() on the writer object to write the dictionary as a row in the CSV file.
Python Program
import csv
my_dict = {
'foo': 12,
'bar': 14
}
with open('data.csv', 'w', newline='') as csv_file:
writer = csv.DictWriter(csv_file, fieldnames=my_dict.keys())
writer.writeheader()
writer.writerow(my_dict)
CopyOutput
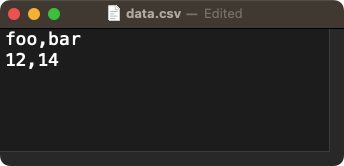
Summary
In this tutorial, we learned how to write a dictionary as a plain text file, how to write a dictionary as a JSON file using json module, and how to write a dictionary as a CSV file using csv module, with help of well detailed Python programs.