Bash Check if String Contains Substring
Bash Check if String Contains Substring
In Bash scripting, checking if a string contains a substring is useful for various string validation and conditional tasks.
Syntax
if [[ $string == *substring* ]]; then
# commands if string contains substring
fi
The basic syntax involves using the == *substring*
pattern within a double-bracket [[ ]]
if statement to check if the string contains the specified substring.
Example Bash Check if String Contains Substring
Let's look at some examples of how to check if a string contains a substring in Bash:
1. Check if a Variable Contains a Substring
This script checks if the variable str
contains the substring 'World' and prints a corresponding message.
#!/bin/bash
str="Hello, World!"
substring="World"
if [[ $str == *$substring* ]]; then
echo "The string contains '$substring'."
else
echo "The string does not contain '$substring'."
fi
In this script, the variable str
is assigned the value 'Hello, World!', and the variable substring
is assigned the value 'World'. The if statement uses the == *$substring*
pattern to check if str
contains substring
. If true, it prints a message indicating that the string contains the substring. Otherwise, it prints a different message.
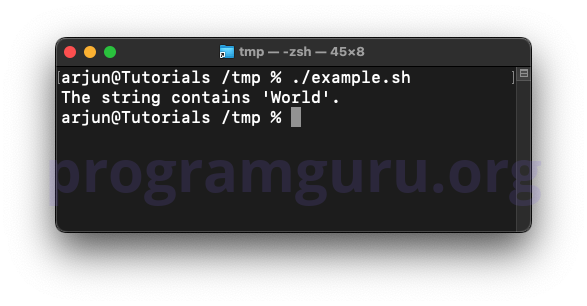
2. Check if a User Input String Contains a Substring
This script prompts the user to enter a string and checks if it contains the substring 'test', then prints a corresponding message.
#!/bin/bash
read -p "Enter a string: " str
substring="test"
if [[ $str == *$substring* ]]; then
echo "The string contains '$substring'."
else
echo "The string does not contain '$substring'."
fi
In this script, the user is prompted to enter a string, which is stored in the variable str
, and the variable substring
is assigned the value 'test'. The if statement uses the == *$substring*
pattern to check if str
contains substring
. If true, it prints a message indicating that the string contains the substring. Otherwise, it prints a different message.
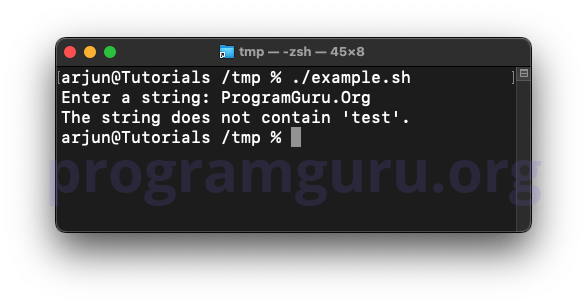
3. Check if a String from a Command Output Contains a Substring
This script checks if the output of a command stored in the variable output
contains the substring 'conf', then prints a corresponding message.
#!/bin/bash
output=$(ls /etc 2>/dev/null)
substring="conf"
if [[ $output == *$substring* ]]; then
echo "The command output contains '$substring'."
else
echo "The command output does not contain '$substring'."
fi
In this script, the variable output
is assigned the result of a command that lists files and directories in the /etc directory, redirecting any errors to /dev/null
. The variable substring
is assigned the value 'conf'. The if statement uses the == *$substring*
pattern to check if output
contains substring
. If true, it prints a message indicating that the command output contains the substring. Otherwise, it prints a different message.
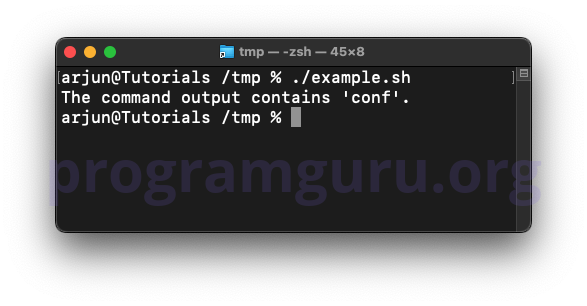
Conclusion
Checking if a string contains a substring in Bash is a fundamental task for string validation and conditional operations in shell scripting. Understanding how to check for substrings can help you manage and validate strings effectively in your scripts.