Bash Iterate Over Array
Bash Iterate Over Array
In Bash scripting, iterating over an array is useful for various tasks that require processing each element of an array.
Syntax
for element in "${array[@]}"; do
# commands to process $element
done
The basic syntax involves using a for
loop to iterate over the elements of the array and process each element.
Example Bash Iterate Over Array
Let's look at some examples of how to iterate over an array in Bash:
1. Print Each Element of an Array
This script initializes an array with elements and prints each element.
#!/bin/bash
array=("element1" "element2" "element3")
for element in "${array[@]}"; do
echo "$element"
done
In this script, the array variable array
is initialized with the elements 'element1', 'element2', and 'element3'. The for
loop iterates over each element in the array and prints it.
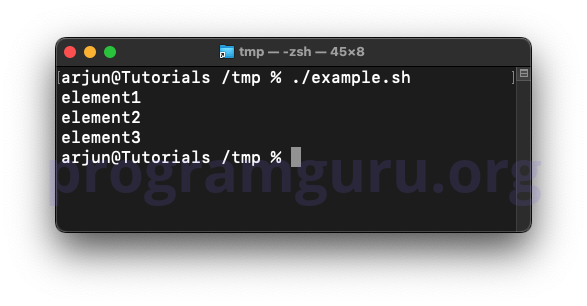
2. Process Each Element of an Array
This script initializes an array with elements, processes each element by converting it to uppercase, and prints the processed elements.
#!/bin/bash
array=("element1" "element2" "element3")
for element in "${array[@]}"; do
processed_element=$(echo "$element" | tr '[:lower:]' '[:upper:]')
echo "$processed_element"
done
In this script, the array variable array
is initialized with the elements 'element1', 'element2', and 'element3'. The for
loop iterates over each element in the array, converts it to uppercase using the tr
command, and prints the processed element.
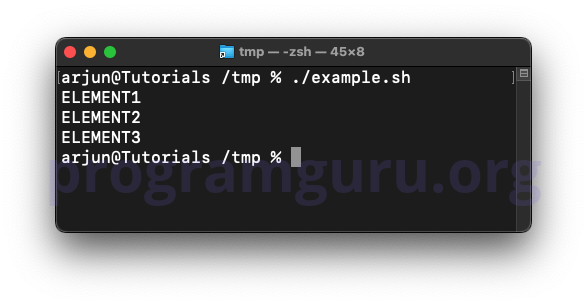
3. Iterate Over an Array with Elements from User Input
This script initializes an array with elements from user input and prints each element.
#!/bin/bash
array=()
while true; do
read -p "Enter an element (or 'done' to finish): " element
if [ "$element" == "done" ]; then
break
fi
array+=("$element")
done
for element in "${array[@]}"; do
echo "$element"
done
In this script, the array variable array
is initialized as an empty array using ()
. The user is prompted to enter elements, which are added to the array using the +=
operator. When the user types 'done', the loop exits. The for
loop then iterates over each element in the array and prints it.
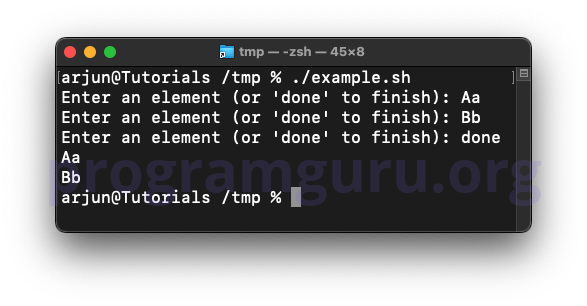
Conclusion
Iterating over an array in Bash is a fundamental task for processing each element of an array in shell scripting. Understanding how to iterate over arrays can help you manage and manipulate arrays effectively in your scripts.