Bash For Loop
Bash For Loop
In Bash scripting, the for loop allows you to iterate over a list of items or a range of numbers, executing a block of commands for each item in the list or number in the range.
Syntax
for variable in list; do
# commands
done
The basic syntax involves using for
followed by a variable name, the in
keyword, a list of items, and the commands to execute for each item in the list. The loop is ended with done
.
Example Bash For Loops
Let's look at some examples of how to use for loops in Bash:
1. For Loop to Iterate Over a List of Strings
This script iterates over a list of names and prints each name.
#!/bin/bash
for name in Alice Bob Charlie; do
echo "Hello, $name!"
done
In this script, the for loop iterates over the list of names 'Alice', 'Bob', and 'Charlie'. For each name, the loop prints a greeting message.
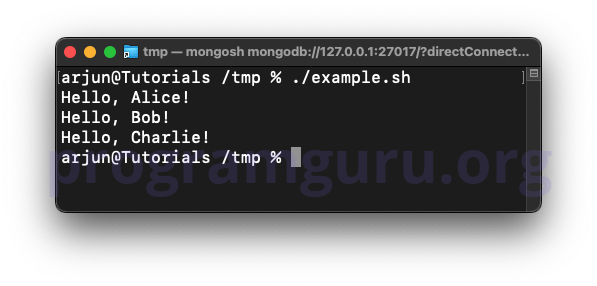
2. For Loop to Iterate Over a Range of Numbers
This script iterates over a range of numbers from 1 to 5 and prints each number.
#!/bin/bash
for number in {1..5}; do
echo "Number: $number"
done
In this script, the for loop iterates over the range of numbers from 1 to 5. For each number, the loop prints the number.
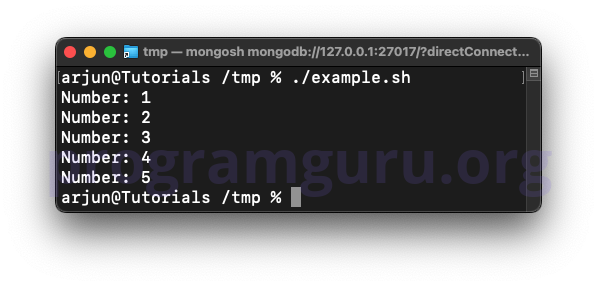
3. For Loop to Iterate Over Files in a Directory
This script iterates over all the files in the current directory and prints the name of each file.
#!/bin/bash
for file in *; do
echo "File: $file"
done
In this script, the for loop iterates over all the files in the current directory (denoted by *
). For each file, the loop prints the file name.
Conclusion
The Bash for loop
is a crucial tool for repetitive tasks in shell scripting. Understanding how to use for loops can help you automate and simplify many tasks in your scripts.