Delete Documents from Collection - PyMongo - Examples
Python MongoDB Delete Documents
You can delete one or more documents from a collection using delete_one() or delete_many() functions respectively.
Delete One Document
delete_one() function can be called on a collection. The function takes query as an argument and deletes only the first and single document that matches the given query.
Example
In the following example, we will delete a single document that matches the given criteria.
Python Program
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
# Use database named "organisation"
mydb = myclient["organisation"]
# Use collection named "developers"
mycol = mydb["developers"]
print('Documents in Collection\n-----------------------')
for doc in mycol.find():
print(doc)
# Query to delete document
query = {'address':'India'}
# Delete one document
mycol.delete_one(query)
print('\nDocuments in Collection after delete_one()\n-----------------------')
for doc in mycol.find():
print(doc)
Output
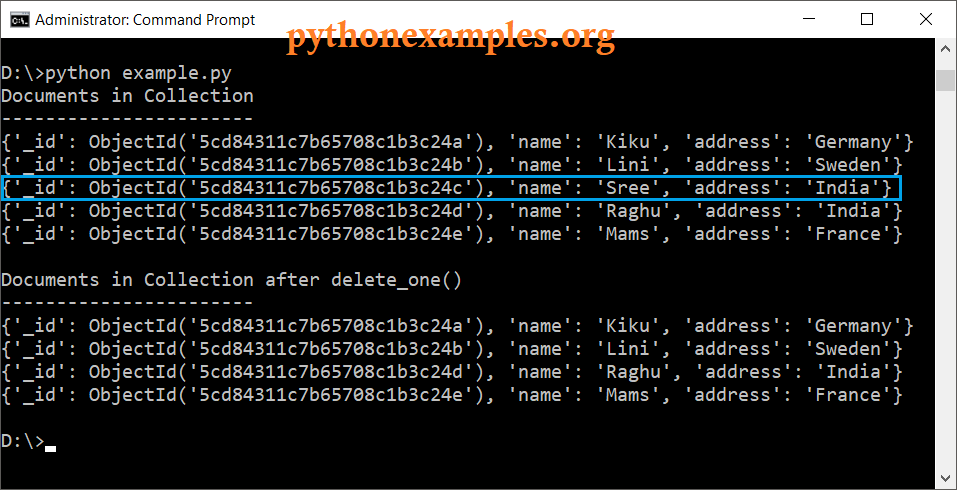
Delete many documents
delete_many() function can be called on a collection. The function takes query as an argument and deletes all the documents that matches the given query.
Example
In the following example, we will delete multiple documents that match the given criteria.
Python Program
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
#use database named "organisation"
mydb = myclient["organisation"]
#use collection named "developers"
mycol = mydb["developers"]
print('Documents in Collection\n-----------------------')
for doc in mycol.find():
print(doc)
#query to delete document
query = {'address':'India'}
#delete many document
mycol.delete_many(query)
print('\nDocuments in Collection after delete_many ()\n-----------------------')
for doc in mycol.find():
print(doc)
Output
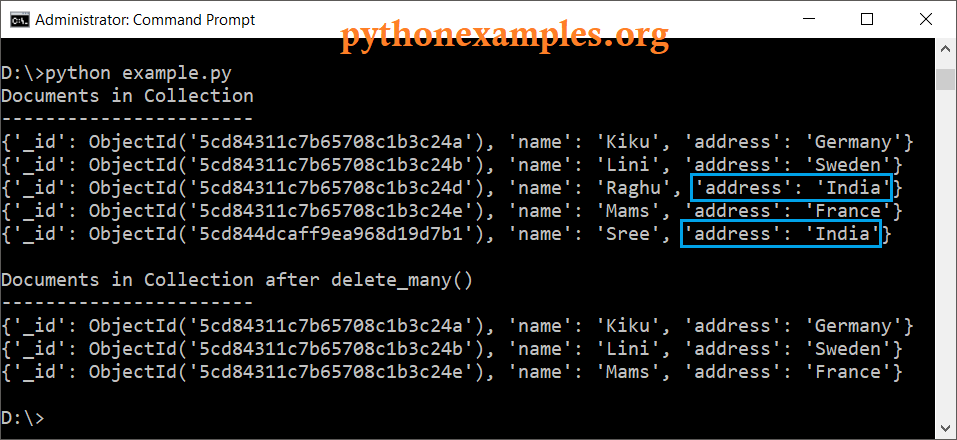
Summary
In this PyMongo Tutorial, we learned how to delete one or more documents from a collection, using delete_one(), and delete_many() functions.