Delete or Drop Collection - PyMongo - Examples
Python MongoDB Delete Collection
To delete a MongoDB Collection in Python language, we shall use PyMongo. Follow these steps to delete a specific MongoDB Collection.
- Create a MongoDB Client to the MongoDB instance.
- Select database using client object. It is assumed that the collection we are going to delete is present in this database.
- Select collection using the database object.
- Use drop() function on the collection object to completely delete the specified collection from the database.
Examples
1. Delete MongoDB collection whose name is "developers"
In the following example, we shall delete developers collection. Also, for understanding, we will print the list of collections present in the database before and after deleting the collection.
Python Program
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
#use database named "organisation"
mydb = myclient["organisation"]
print("List of collections before deletion\n--------------------------")
for x in mydb.list_collection_names():
print(x)
#get collection named "developers"
mycol = mydb["developers"]
#delete or drop collection
mycol.drop()
print("\nList of collections after deletion\n--------------------------")
for x in mydb.list_collection_names():
print(x)
Output
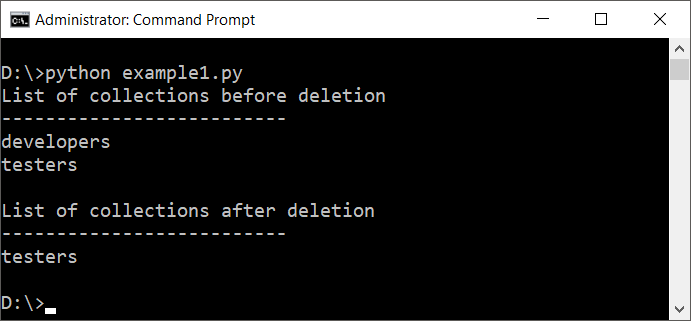
Summary
In this PyMongo Tutorial, we learned how to delete a MongoDB collection by name using drop() function.