PyMongo – Insert Document to MongoDB using Python
You can insert one or more documents into a MongoDB using insert_one() or insert_many() methods of the Collection class.
In this tutorial, you shall learn the steps to insert document(s) to MongoDB using insert_one() or insert_many() functions, with examples.
1. Insert single document to MongoDB database
To insert a document to MongoDB Collection in Python language, we use PyMongo and follow these steps.
- Create a client to the MongoDB instance.
- Provide the name of the database to the client. It returns a reference to the Database.
- Provide your new collection name as index to the database reference.
It returns a reference to the Collection. - Use insert_one() method on the collection and pass a dictionary as argument to the function.
A quick code snippet to insert one document to MongoDB using Python is:
x = mycollection.insert_one({'key1':'value1', 'key2':'value2'})
Example
In the following example, we are inserting a document to the collection named “developers”.
Python Program
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
#use database named "organisation"
mydb = myclient["organisation"]
#use collection named "developers"
mycol = mydb["developers"]
#dictionary as a document
dict = { "name": "Kiku", "address": "Germany" }
#insert a document to the collection
x = mycol.insert_one(dict)
#id returned by insert_one
print("Document inserted with id: ", x.inserted_id)
print("\nDocuments in developers collection\n----------------------------------")
#print all the documents in the collection
for x in mycol.find():
print(x)
Output
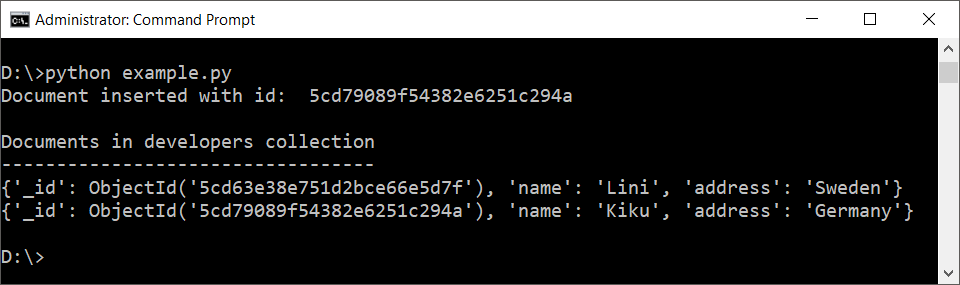
Note: Make sure that you provide the name of the database and collection correctly. Otherwise, a new database or collection would be created if it does not exist already. And all this happens without any warning that the database or collection is not present.
2. Insert Multiple Documents into MongoDB Collection
You can use insert_many() function to insert a list of documents. insert_many() takes Python List as an argument and inserts those documents in the collection.
Example
In the following example, we have selected developers collection of organisation database. Then we initialized a list with multiple documents. We passed the list to insert_many() function and it does the job.
Python Program
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
#use database named "organisation"
mydb = myclient["organisation"]
#use collection named "developers"
mycol = mydb["developers"]
#list of documents
list = [{ "name": "Kiku", "address": "Germany" },
{ "name": "Lini", "address": "Sweden" },
{ "name": "Sree", "address": "India" },
{ "name": "Raghu", "address": "India" },
{ "name": "Mams", "address": "France" }]
#insert multiple documents
x = mycol.insert_many(list)
print('ids of inserted documents\n---------------------')
for id in x.inserted_ids:
print(id)
insert_many() function returns the list of document ids that are inserted to the collection. You can store them in a variable and access them.
Output
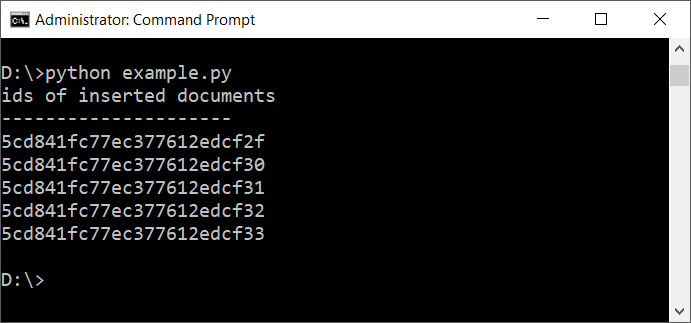
Summary
In this PyMongo Tutorial, we learned how to insert a document to a MongoDB database using insert_one() or insert_many() functions.