Get Collection Names of Database - PyMongo - Examples
List Collection Names of MongoDB Database
To list collection names present in a MongoDB database, follow these steps:
- Create a client to the MongoDB instance.
- Select a database from the client. This returns a reference to the database.
- Call the function
list_collection_names()
on the database reference. - The function returns an iterator. Use a
for
loop to iterate through the list of collections.
Examples
1. Get List of MongoDB Collections
The following Python program lists the collection names present in a MongoDB database.
Python Program
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
# Use database "organisation"
mydb = myclient['organisation']
print("List of collections\n--------------------")
# List the collections
for coll in mydb.list_collection_names():
print(coll)
Explanation:
- We import the
pymongo
library and establish a connection to the local MongoDB instance usingMongoClient
. - We select the
organisation
database usingmyclient['organisation']
. - We call
list_collection_names()
on the selected database referencemydb
to retrieve the list of collections. - The function returns an iterator, which we loop over using a
for
loop and print each collection name.
Output:
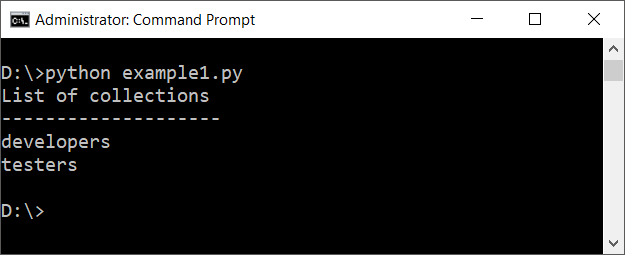
2. Get Collection Names in a Remote MongoDB Instance
In this example, we connect to a remote MongoDB instance and list the collections from a database.
Python Program
import pymongo
myclient = pymongo.MongoClient("mongodb://remote_host:27017/")
# Use database "company"
mydb = myclient['company']
print("List of collections in 'company' database\n----------------------------------")
# List the collections
for coll in mydb.list_collection_names():
print(coll)
Explanation:
- We connect to a remote MongoDB instance by providing the host URL
mongodb://remote_host:27017/
. - We select the
company
database from the remote instance usingmyclient['company']
. - We then call
list_collection_names()
on thecompany
database and print the collection names in that database.
3. Handle Empty Databases or Databases with No Collections
In some cases, a database might not have any collections. Here's an example to handle such cases gracefully.
Python Program
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
# Use an empty database "emptyDB"
mydb = myclient['emptyDB']
# List collections, check if any exist
collections = mydb.list_collection_names()
if collections:
print("Collections in 'emptyDB':", collections)
else:
print("No collections found in 'emptyDB'.")
Explanation:
- We select a database named
emptyDB
which has no collections. - We use
list_collection_names()
to get the list of collections, and then check if any collections exist. - If the list is empty, we print a message indicating no collections were found.
Output:
No collections found in 'emptyDB'.
Summary
In this PyMongo Tutorial, we learned how to get the list of collections in a MongoDB database using list_collection_names()
function, with examples demonstrating:
- Listing collection names in a local MongoDB database.
- Listing collections in a remote MongoDB instance.
- Handling empty databases with no collections.