List Database Names - PyMongo - Examples
PyMongo List Database Names
To list databases present in a MongoDB instance,
- Create a client to the MongoDB instance.
- Using the client, call the
list_databases()
function. - The function returns an iterator. Use a For loop to iterate through the list of databases.
Examples
1. List Databases Present in MongoDB Instance
In the following program, we connect to the MongoDB instance running locally. We then call list_databases()
on the connection object, and using a For loop, print the database details.
Python Program
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
for db in myclient.list_databases():
print(db)
Explanation:
- We import the
pymongo
library, which is used to interact with MongoDB in Python. - We create a client object
myclient
to connect to the MongoDB instance running on localhost at port 27017. - We call the
list_databases()
method on themyclient
object to retrieve a list of all databases present in the MongoDB instance. - Using a For loop, we iterate through each database and print its details.
Output
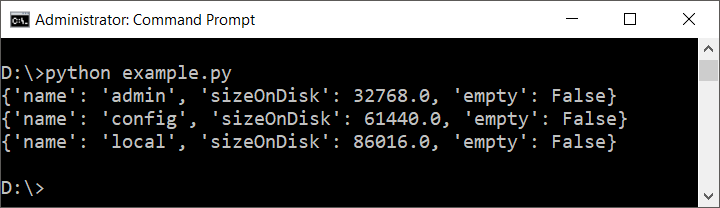
2. List Databases with Additional Information
In this example, we will list the databases and display additional information like the size of the database. This can be useful when you need more details about each database.
Python Program
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
for db in myclient.list_databases():
print(f"Database Name: {db['name']} - Size: {db['sizeOnDisk']} bytes")
Explanation:
- We use the same MongoDB client connection.
- For each database, we access the name and size of the database by using the dictionary keys
'name'
and'sizeOnDisk'
. - We print the database name along with its size on disk in bytes.
Output

3. List Databases with a Filter (Database Type)
MongoDB does not provide a built-in method to filter databases based on types or conditions. However, you can manually filter the databases using Python's built-in functionalities.
In this example, we will list only databases whose names contain the word 'test'
.
Python Program
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
for db in myclient.list_databases():
if 'test' in db['name']:
print(db['name'])
Explanation:
- After connecting to MongoDB, we use a for loop to iterate through the list of databases.
- We check if the word
'test'
is in the name of each database using a simple conditionalif 'test' in db['name']
. - If the condition is true, we print the database name.
Output

Summary
In this PyMongo Tutorial, we learned how to get the list of databases present in a MongoDB instance using the list_databases()
function, with examples. We also explored how to retrieve additional information about the databases and filter the list based on specific criteria.