Fibonacci Series using For Loop
Fibonacci Series in Python using For Loop
In this tutorial, we will write a Python program to print Fibonacci series, using for loop.
Fibonacci Series is a series that starts with the elements 0 and 1, and continue with next element in the series as sum of its previous two numbers.
Fibonacci Series Program
In this example, we read a number from user, N
as input. N represents the number of elements of Fibonacci Series to be generated and print to the console.
Python Program
N = int(input("Number of elements in Fibonacci Series, N, (N>=2) : "))
#initialize the list with starting elements: 0, 1
fibonacciSeries = [0,1]
if N>2:
for i in range(2, N):
#next elment in series = sum of its previous two numbers
nextElement = fibonacciSeries[i-1] + fibonacciSeries[i-2]
#append the element to the series
fibonacciSeries.append(nextElement)
print(fibonacciSeries)
Output
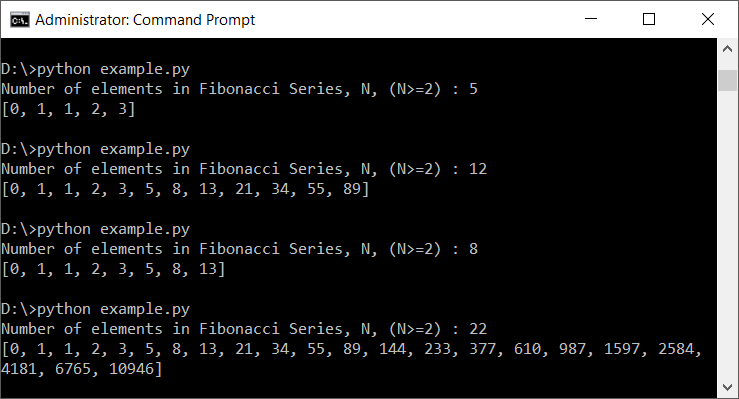
We store the fibonacci series in a Python List with initial values of [0, 1]. As and when we compute the next element in the series, we append that element to the list. We shall loop this process for a range of [2, N], where N is obtained from the user which represents number of elements to be generated in Fibonacci Series.
Summary
In this tutorial of Python Examples, we learned how to generate a Fibonacci series in Python using for loop.