Pillow – Create GIF
To create a GIF from a list of images with Pillow library in Python, you can use Image.save() function.
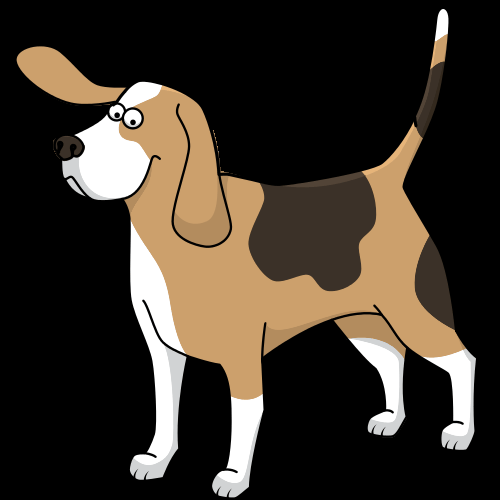
Steps to create a GIF image
Steps to convert a given color image to a grayscale image.
- Import Image module from Pillow library.
- Create a list of images that you want to include in the GIF. These are the list of image objects.
- Save the first image as a GIF file using PIL.Image.save(), and set the save_all parameter to True. Pass the rest of images as a list to append_images parameter. Set the duration (in milliseconds) for each image in the animation, and loop=0 for looping indefinitely.
Examples
1. Convert list of images to GIF
In the following example, we take three images of size 500×500, and convert them into a GIF animation.
Python Program
from PIL import Image
# Take list of paths for images
image_path_list = ['dog-1.jpg', 'dog-2.jpg', 'dog-3.jpg']
# Create a list of image objects
image_list = [Image.open(file) for file in image_path_list]
# Save the first image as a GIF file
image_list[0].save(
'animation.gif',
save_all=True,
append_images=image_list[1:], # append rest of the images
duration=1000, # in milliseconds
loop=0)
Original Images
dog-1.jpg
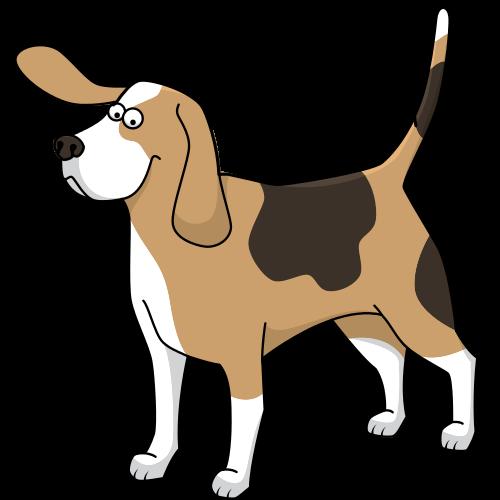
dog-2.jpg
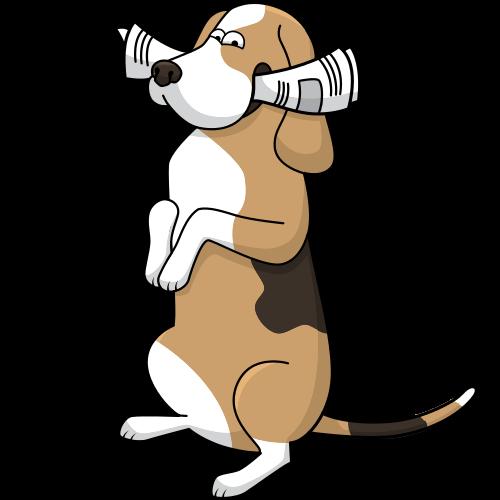
dog-3.jpg
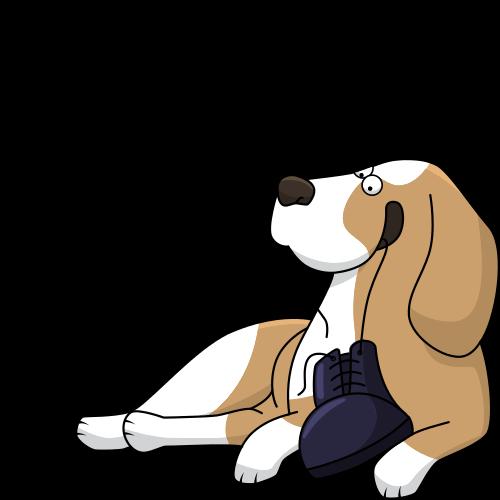
Resulting GIF image
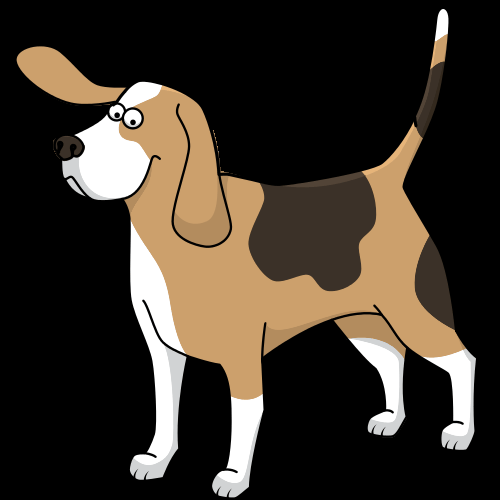
Summary
In this Python Pillow Tutorial, we learned how to create a GIF image using PIL.Image.save(), with the help of examples.