How to Read Input from Console in Python?
Python - Read input from console
To read input from user, we can use Python builtin function input().
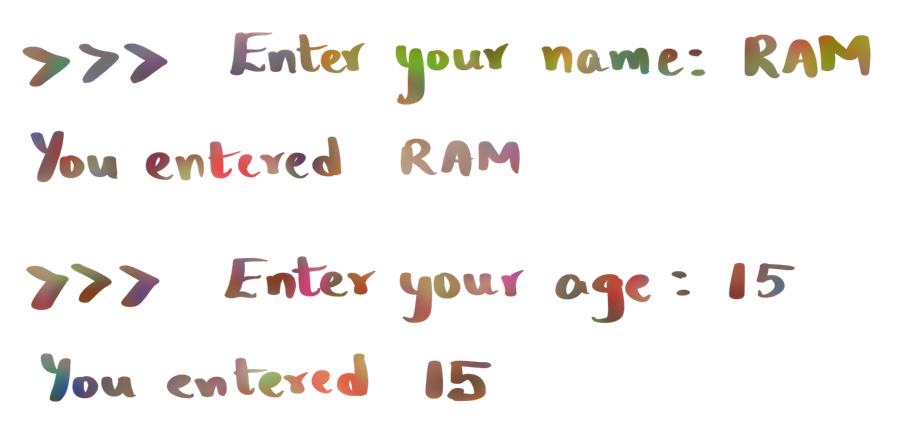
input() builtin function is used to read a string from standard input. The standard input in most cases would be your keyboard.
Syntax
x = input(prompt)
where prompt
is a string.
input() function returns the string keyed in by user in the standard input.
Examples
1. Read string value from user
In this example, we shall read a string from user using input() function.
Python Program
x = input('Enter a value : ')
print('You entered :', x)
Run the program
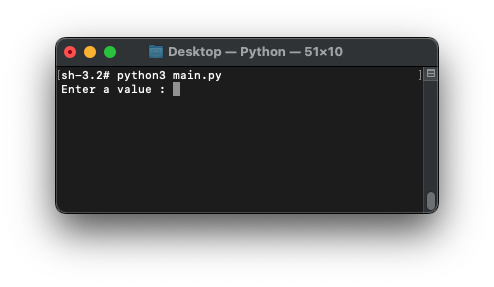
Enter input
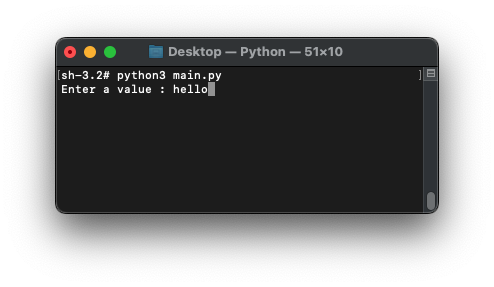
Hit Enter or Return key
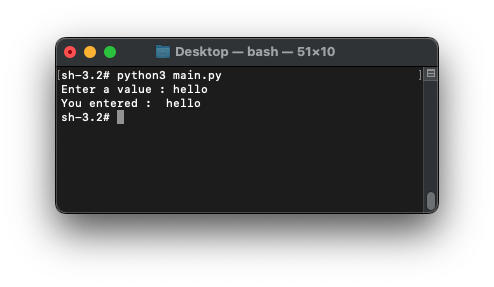
2. Read number using input()
By default, input() function returns a string. If you would like to read a number from user, you can typecast the string to int, float or complex, using int(), float() and complex() functions respectively.
In the following program, we shall read input from user and convert them to integer, float and complex.
Python Program
x = int(input('Enter an integer : '))
print('You entered : ', x)
x = float(input('Enter a float : '))
print('You entered : ', x)
Output
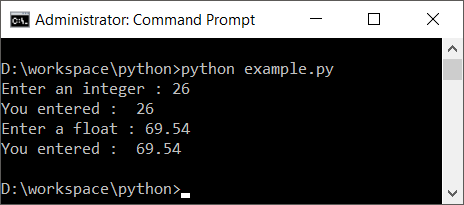
3. input() - without prompt message
Prompt string is an optional argument to input() function.
In this example, let us not provide a string argument to input() and read a string from user.
Python Program
x = input()
print('You entered : ', x)
Output
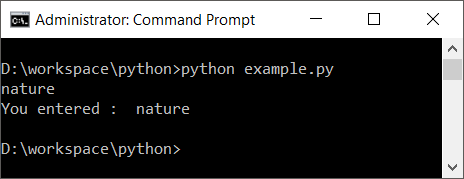
Summary
In this tutorial of Python Examples, we learned how to read input or string from user via system standard input.