Open URL in Chrome Browser from Python Application
Python - Open URL in Chrome Browser
You can open URL in a browser and also specify to open the URL in Chrome browser specifically.
To open URL in a browser, we will use webbrowser
python module.
Step-by-step Process
Follow these steps in your Python Application to open URL in Chrome Browser.
- Register the browser type name using
webbrowser.register()
. Also provide the browser executable file path. - Get the controller object for the browser
using webbrowser.get()
and Open URL usingopen()
.
Example 1: Open URL in Google Chrome
In this example, we will open the URL https://pythonexamples.org in Chrome browser.
Python Program
import webbrowser
url = 'https://pythonexamples.org'
webbrowser.register('chrome',
None,
webbrowser.BackgroundBrowser("C://Program Files (x86)//Google//Chrome//Application//chrome.exe"))
webbrowser.get('chrome').open(url)
webbrowser.get('chrome').open(url)
opens a new window if already not opened, or opens in a new tab if Chrome is already opened in your PC.
Output
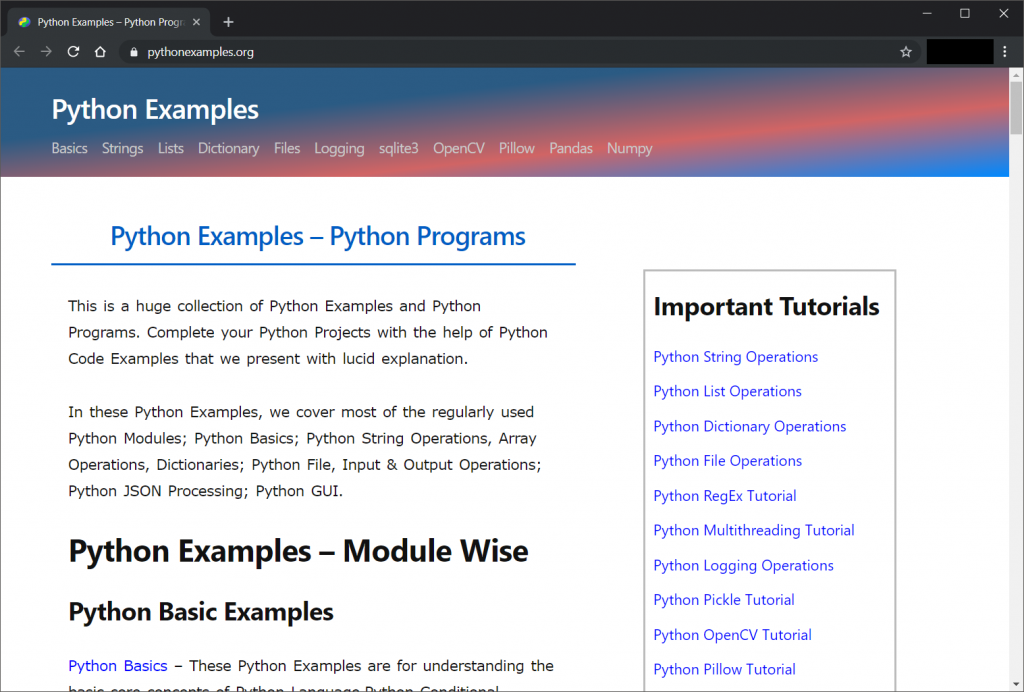
Example 2: Open URL in a new Google Chrome Window
At times, there would already be a Chrome browser opened and you would like to open this URL in a new window.
In this example, we will try to open the URL https://pythonexamples.org in a new Chrome browser.
Python Program
import webbrowser
url = 'https://pythonexamples.org'
webbrowser.register('chrome',
None,
webbrowser.BackgroundBrowser("C://Program Files (x86)//Google//Chrome//Application//chrome.exe"))
webbrowser.get('chrome').open_new(url)
open_new()
tries to open a new Chrome browser window, only if possible.
Summary
In this tutorial of Python Examples, we learned how to open a URL in Chrome browser.