Python Pillow - Add Watermark to Image
Python Pillow - Add Watermark to Image
You can add a transparent watermark to an image using Pillow library in Python.
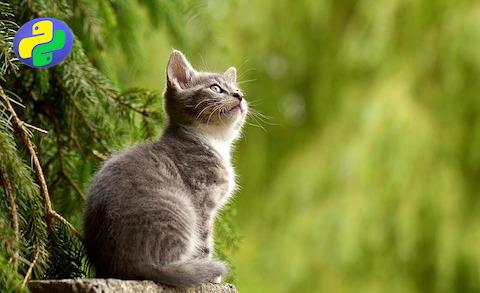
Watermarks are generally added to images to specify the branding, or specify copyright information, or in such cases.
To add a watermark to an image using the Python Pillow library (PIL), you can overlay a transparent watermark image on top of the original image at specific position using Image.paste().
Let us go through some examples. The following are the input images.
Original Image
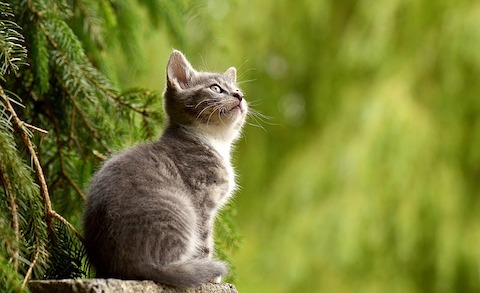
Watermark Image

1. Add watermark to image using Image.paste() in Pillow
We are given two images: input_image.jpg
, and watermark.jpg
. We have to add the water mark image to the original image input_image.jpg at top left corner, precisely at location (10, 10).
Steps
- Open original image using Image.open().
original_image = Image.open('input_image.jpg')
- Open the watermark image using Image.open(). You may replace
'watermark.png'
with your watermark image file.
watermark = Image.open('watermark.png')
- Define the position where you want to place the watermark. Adjust these coordinates to place the watermark as desired. This position must be a tuple of two integers, specifying the distance (in pixels) from top and left respectively.
position = (10, 10)
- Paste the watermark onto the original image using Image.paste().
original_image.paste(watermark, position, watermark)
- Save the modified image object to local file, say
output_image.jpg
.
original_image.save('output_image.jpg')
- Finally, close both the original image and watermark image.
original_image.save('output_image.jpg')
Program
The complete program to add a watermark to an image using Image.paste() is given below.
Python Program
from PIL import Image
# Open the original image
original_image = Image.open('input_image.jpg')
# Open the watermark image
watermark = Image.open('watermark.png')
# Coordinates to place the watermark
position = (10, 10)
# Add watermark
original_image.paste(watermark, position, watermark)
# Save the image with the watermark
original_image.save('output_image.jpg')
# Close both images
original_image.close()
watermark.close()
Output Image
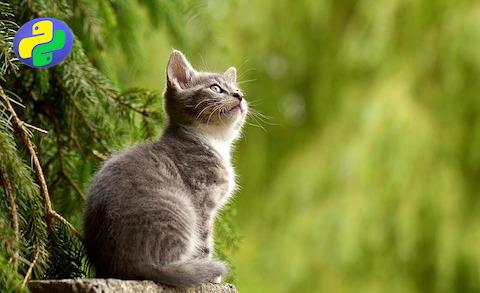
2. Set transparency to watermark image
In the previous example, we have seen how to add a watermark image to an original image. Now, we take it a step further by adding transparency to the watermark image.
The additional step would be, after reading the watermark image, take a transparency value that can range from 0 to 255 where 0 represents fully transparent watermark, and 255 represents fully opaque watermark. And use this transparency value for the alpha channel of the watermark image.
Program
The complete program to add a watermark with a specific transparency to an image is given below.
Python Program
from PIL import Image
# Open the original image
original_image = Image.open('input_image.jpg')
# Open the watermark image
watermark = Image.open('watermark.png')
# Transparency level (0 for fully transparent, 255 for fully opaque)
transparency = 150
# Make the watermark image transparent by adjusting the alpha channel
watermark = watermark.convert('RGBA')
watermark_with_transparency = Image.new('RGBA', watermark.size)
for x in range(watermark.width):
for y in range(watermark.height):
r, g, b, a = watermark.getpixel((x, y))
if not (r == 0 and g == 0 and b == 0):
watermark_with_transparency.putpixel((x, y), (r, g, b, transparency))
# Coordinates to place the watermark
position = (10, 10)
# Add watermark
original_image.paste(watermark_with_transparency, position, watermark_with_transparency)
# Save the image with the watermark
original_image.save('output_image.jpg')
# Close both images
original_image.close()
watermark.close()
Output Image
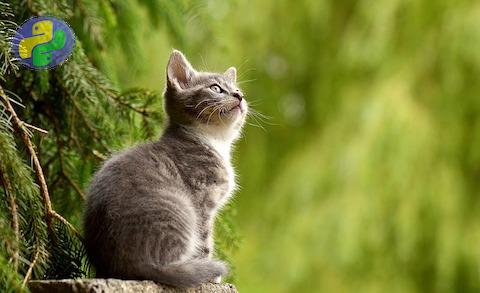
Summary
In this Python Pillow tutorial, we learned how to add watermark to an image using Pillow library in Python, with examples. We have taken an input image, a transparent watermark image, and then added the watermark image to the input image.